Exploring STC 8051 Microcontrollers – Coding
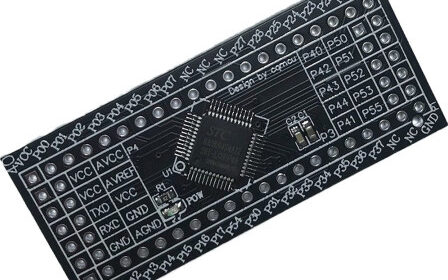
|
Timer 1 as a Counter
Apart from timing events, the other role of timers is to count pulses. Pulse counting has number of applications like motor speed control, object counting, frequency measurements, encoder counting, etc.
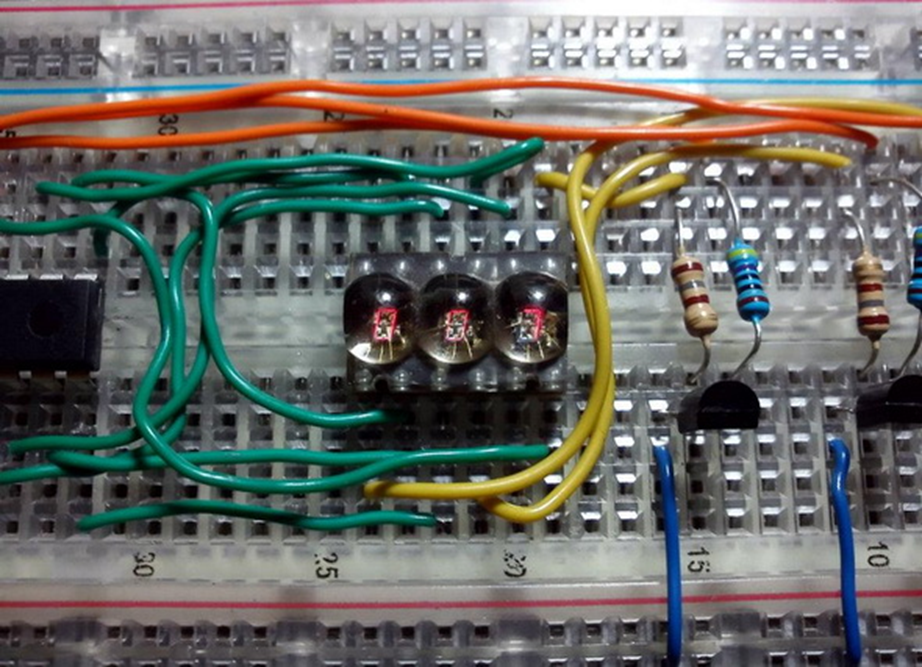
Code
#include "STC8xxx.h"
#include "BSP.h"
void setup(void);
void main(void)
{
setup();
while(1)
{
if(TMR1_get_counter() >= 32768)
{
P55_high;
}
else
{
P55_low;
}
};
}
void setup(void)
{
CLK_set_sys_clk(IRC_24M, 2, MCLK_SYSCLK_no_output, MCLK_out_P54);
P34_push_pull_mode;
P35_input_mode;
P55_open_drain_mode;
TMR1_setup(TMR1_16_bit_non_auto_reload, \
TMR1_ext_T1_P35_clk, \
TMR1_clk_prescalar_12T, \
TMR1_int_gate, \
TMR1_P34_clk_out);
TMR1_load_counter_8(0x00, 0x00);
TMR1_start;
}
Schematic
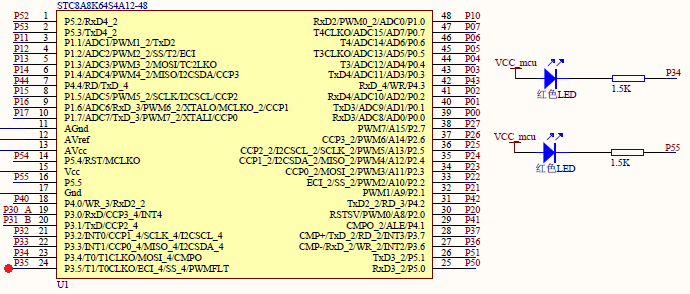
Explanation
This example is same as the previous timer example, the only difference is the clock source of the timer involved here. Timer 1 is used and it is externally clocked by using a signal generator. In this example, the blinking rate of the onboard LED changes with the clock speed of the timer.
First let’s see how the timer is set up.
TMR1_setup(TMR1_16_bit_non_auto_reload, \
TMR1_ext_T1_P35_clk, \
TMR1_clk_prescalar_12T, \
TMR1_int_gate, \
TMR1_P34_clk_out);
TMR1_load_counter_8(0x00, 0x00);
TMR1_start;
Here, we can, firstly, see that the timer is set in non-auto reload mode. This means that timer needs periodic reloads after overflow or else it will restart counting from 0. In our case, this won’t matter because we want the timer to count from 0 after overflow. Secondly, we can see that the timer is externally clocked and this is achieved by feeding clock pulses to P3.5 (T1) GPIO pin. Since the timer is externally clocked, it doesn’t matter what system clock settings are. The next thing to note is the timer’s clock prescalar. This is needed because we want to visually see the outcome with LEDs. If the LEDs blink too fast, we won’t not be able to realize what is going on. Lastly, internal gating is used because we don’t the timer to depend on other hardware events and we want to get timer output. Timer 1 will toggle the logic state of P3.4 (T1CLKO) GPIO pin when it overflows. Both GPIO pins P3.4 and P3.5 need to configured.
P34_push_pull_mode;
P35_input_mode;
State of P5.5 LED changes according to the value of timer’s internal counter. For one half of the 16-bit count the LED is held low and for the other half it is held high.
if(TMR1_get_counter() >= 32768)
{
P55_high;
}
else
{
P55_low;
}
Thus, in the main, this is only job done. Rate of blinking depends on external clock speed. The higher the clock frequency, the higher is the blinking rate.
Demo
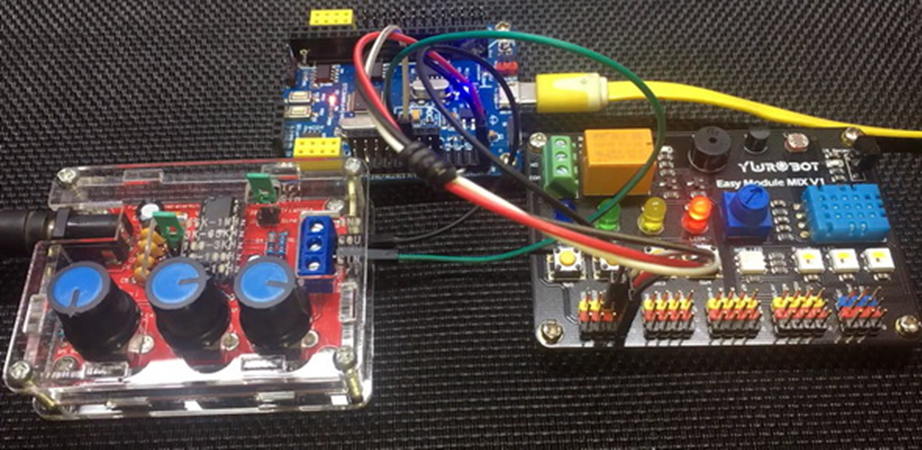
|
hello.
this is a very good effort to document all and still share with us. thank you very much.
I have one doubt . which programming tool are you using ?
Hi, I am trying to understand the STC15w408as chip, and found this site after weeks of searching for something that sets the output of the GPIO pins to a different state. I have a the 28 pin stc15w and have connected it up with a FTDI board and can write to it using PlatformIO. The thing is, the GPIO ports if just switched on or do a reset they are in the HIGH state and I am trying to make them LOW when you do a reset.
Is your BSP code doing this and for what port or GPIO pin is it setting? I could change your P52 and P55 in your SETUP to the GPIO pins on my development board but not under standing the BSP Code.
Wonder if you get this post? but any help would be gratefully received.
Hi,
How Purchase the development board. Please,give the purchase link for this Development board.
https://www.alibaba.com/product-detail/Development-board-1T-STC8A8K64S4A12-single-chip_62391507065.html
https://world.taobao.com/item/600882463994.htm
https://www.amazon.ca/STC8A8K64S4A12-Development-Controller-Module-Minimal/dp/B08D3Y3R6T
How To read and write string data using IAP into memory
void IAP_erase(unsigned int address)
{
IAP_CONTR = 0x80; //?? IAP
IAP_TPS = 12;
// IAP_CONTR = IAP_WT;
IAP_CMD = IAP_erase_command;
IAP_address(address);
IAP_trigger;
_nop_();
_nop_();
_nop_();
IAP_clear;
}
void IAP_send_string(unsigned int uc_send_addr,unsigned char *uca_send_string,unsigned int uc_number_of_bytes)
{
unsigned int buff_cntr=0;
do
{
IAP_CONTR = 0x80; //?? ISP/IAP ??
IAP_TPS = (unsigned char)(11509200 / 1000000L); //??????
IAP_CMD = IAP_write_command;
// IAP_CMD = IAP_write_command;
IAP_ADDRH = uc_send_addr / 256; //??????(??????????????)
IAP_ADDRL = uc_send_addr % 256; //??????
IAP_DATA = uca_send_string[buff_cntr]; //???? ISP_DATA,????????????
IAP_trigger;//IAP_TRIG();
_nop_();
_nop_();
_nop_();
uc_send_addr++;
// uca_send_string++;
buff_cntr++;
IAP_clear;
delay_ms(8);
}while(–uc_number_of_bytes);
}
void IAP_read_string(unsigned int uc_read_addr,unsigned char *data_read,unsigned int uc_number_of_bytes)
{
unsigned int buff_cntr=0;
do{
IAP_CONTR = 0x80; //?? ISP/IAP ??
IAP_TPS = (unsigned char)(11059200 / 1000000L); //??????
IAP_CMD = IAP_read_command;
// IAP_CMD = IAP_read_command;
IAP_ADDRH = uc_read_addr / 256; //??????(??????????????)
IAP_ADDRL = uc_read_addr % 256; //??????
IAP_trigger;//IAP_TRIG(); //?? 5AH,?? A5H ? ISP/IAP ?????,
//???????
//?? A5H ?, ISP/IAP ?????????
//CPU ?? IAP ???,?????????
_nop_();
_nop_();
_nop_();
data_read[buff_cntr] = IAP_DATA; //???????
uc_read_addr++;
// data_read++;
buff_cntr++;
IAP_clear;
delay_ms(8);
}while(–uc_number_of_bytes);
}
stores only last byte to all bytes of flash memory sector… memory sector selected is 0xF600
Hi, I am using STC MCU since 10 years. Tech support is ZERO. but they are low cost, very stable. Now I have a problem when the chip that I used is obsolete. Now start to use STC8C2K64S4-28I-LQFP32 but no stc8Cxx.h file, I am using stc8Hxx.h file which compiles but in some stage freeze, the existing firmware. With stc8hxx.h file I can compile STC8F2K64S4-28I-LQFP32 and works not bad
.
I wrote them many times for the stc8Cxx.h file never got answer. Where Can I find that file?
Thank you
Give me detail 8f2k64s281MCU read and write programmer
Give me detail 8f2k64s281reed and write programmer distal
Hi. Can you explain how to use I2C in the slave mode ?
I tried STC8G1K08A i2c in slave mode. Doesn’t work (no response). It does not enter interrupt, even on a start condition (everything according to the code in the documentation). I also tried master mode – it works.
Thanks for these tutorials. I’m getting back into STCmicro coding now, having left them alone for the past several years. Back then I only used the STC89C52RC (and C54RD) but this time I’m also using the more powerful STC15 and STC8 types. Your blogs provide a wealth of useful information.
Hello,
You have done great job with all these tutorials. I am an electronics engineer trying to learn some new stuff. I am located in Greece , Europe and I would like to purchase the development board that you are using and download some datasheets in English if possible but I cannot find them anywhere. Could you please help me?
I suggest you buy from AliExpress or similar platform that is available in your country…. You can find the English datasheet here. English documentation can be found in STC’s official websites such as this one….
Thank you very much for your help!!!
i always get excited when you release new tutorials ,you are really doing a great job i wish i could write code and develop libraries like you.
Well, this is very nice and thorough tutorial indeed, many thanks!
Unfortunately I doubt there is good any reason to learn the STC platform beyond curiosity.
The STC 8051, although pretty evolved from the original 8051 ISA, does not offer anything crucial to justify the relatively high price of these micros and development tools along with certain cumbersomeness of this ancient platform.
They simply can not compete even with the legacy Cortex M0 in any way. I am even not aware about any affordable debugger/emulator for them.
All in all, I would never recommend anybody to start learning/using any 8051 without some very good reason to do so.