Exploring STC 8051 Microcontrollers – Coding
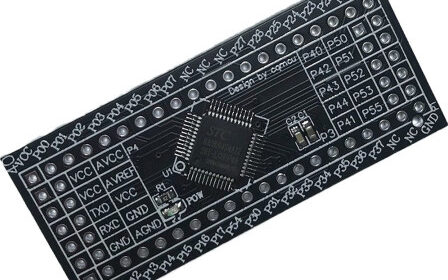
|
Using Multi Channel Enhanced PWM to Drive an RGB LED
Since we now have seen how to use single channel enhanced PWM, we must also see how to use multichannel PWM. Multi-channel PWM has many applications. For example, multi-channel PWM is used in SMPSs, inverters, motor controllers, LED drivers, etc.
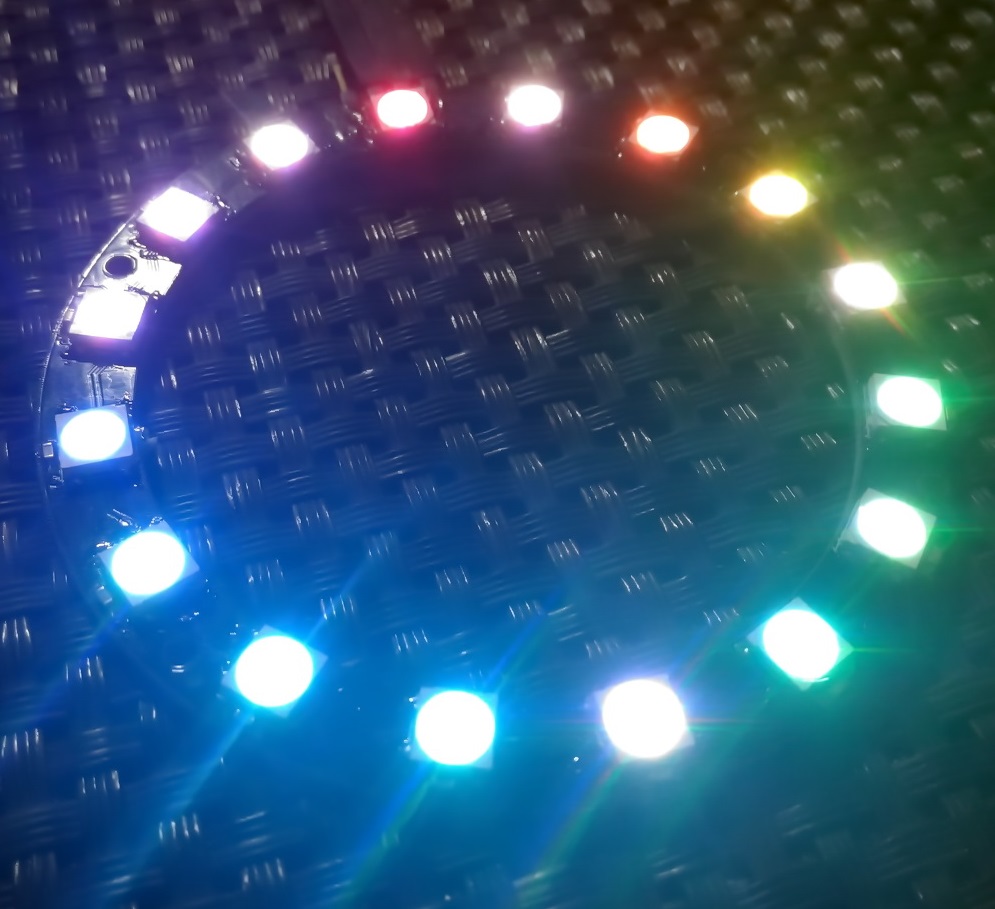
Code
#include "STC8xxx.h"
#include "BSP.h"
#define steps 33
void setup(void);
void PWM_idle(void);
void main(void)
{
signed char i = 0x00;
signed char j = 0x00;
const unsigned int duty1_value[steps] = {0, 950, 1892, 2817, 3717, 4582, 5407, 6182, 6901, 7558, 8146,
8660, 9096, 9450, 9718, 9898, 9988, 9995, 9897, 9717, 9449, 9095,
8658, 8144, 7555, 6898, 6179, 5403, 4579, 3713, 2813, 1888 ,946};
const unsigned int duty2_value[steps] = {8658, 8144, 7555, 6898, 6179, 5403, 4579, 3713, 2813, 1888 ,946,
0, 950, 1892, 2817, 3717, 4582, 5407, 6182, 6901, 7558, 8146,
8660, 9096, 9450, 9718, 9898, 9988, 9995, 9897, 9717, 9449, 9095};
const unsigned int duty3_value[steps] = {8660, 9096, 9450, 9718, 9898, 9988, 9995, 9897, 9717, 9449, 9095,
8658, 8144, 7555, 6898, 6179, 5403, 4579, 3713, 2813, 1888 ,946,
0, 950, 1892, 2817, 3717, 4582, 5407, 6182, 6901, 7558, 8146};
setup();
while(1)
{
for(j = 0; j < 6; j++)
{
for(i = 0; i < steps; i++)
{
PWM_set_PWM0_T2(duty1_value[i]);
PWM_set_PWM1_T2(duty2_value[i]);
PWM_set_PWM2_T2(duty3_value[i]);
delay_ms(60);
}
}
PWM_idle();
for(j = 0; j < 6; j++)
{
for(i = 0; i < steps; i++)
{
PWM_set_PWM0_T2(duty1_value[i]);
PWM_set_PWM1_T2(duty1_value[i]);
PWM_set_PWM2_T2(duty1_value[i]);
delay_ms(60);
}
}
PWM_idle();
};
}
void setup(void)
{
CLK_set_sys_clk(IRC_24M, 2, MCLK_SYSCLK_no_output, MCLK_out_P54);
PWM_clk_set(PWM_clk_sys_PS, PWM_clk_ps_sys_clk_div_2);
PWM_set_counter(10000);
PWM0_setup(PWM_pin_is_PWM_output, \
PWM_init_lvl_low, \
PWM_0_pin_P10, \
PWM_level_normal);
PWM1_setup(PWM_pin_is_PWM_output, \
PWM_init_lvl_low, \
PWM_1_pin_P11, \
PWM_level_normal);
PWM2_setup(PWM_pin_is_PWM_output, \
PWM_init_lvl_low, \
PWM_2_pin_P12, \
PWM_level_normal);
PWM_set_PWM0_T1(0);
PWM_set_PWM0_T2(0);
PWM_set_PWM1_T1(0);
PWM_set_PWM1_T2(0);
PWM_set_PWM2_T1(0);
PWM_set_PWM2_T2(0);
PWM_start_counter;
}
void PWM_idle(void)
{
PWM0_hold_level(PWM_HLD_L_low);
PWM1_hold_level(PWM_HLD_L_low);
PWM2_hold_level(PWM_HLD_L_low);
delay_ms(100);
PWM0_hold_level(PWM_level_normal);
PWM1_hold_level(PWM_level_normal);
PWM2_hold_level(PWM_level_normal);
delay_ms(100);
}
Schematic
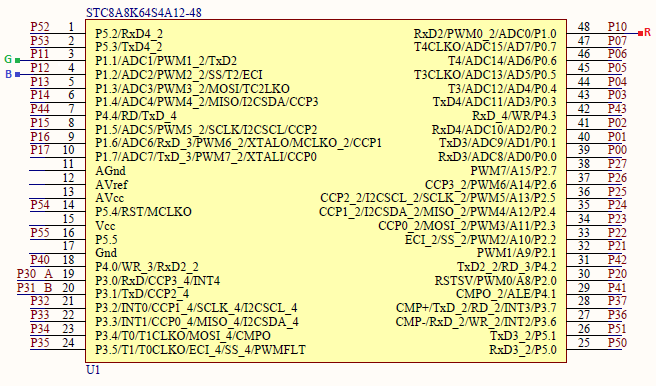
Explanation
In this demo, a RGB LED is lit with multi-channel PWM. This results in smooth color shifting of the LED. The concepts are same as that of single channel PWM example.
The system clock is set to 12MHz.
CLK_set_sys_clk(IRC_24M, 2, MCLK_SYSCLK_no_output, MCLK_out_P54);
The PWM frequency is set to 600 Hz.

Since we are using a RGB LED, we need three PWM channels and so their GPIOs are also set. Lastly, the initial duty cycle is set 0 and the PWM counter is started.
PWM_clk_set(PWM_clk_sys_PS, PWM_clk_ps_sys_clk_div_2);
PWM_set_counter(10000);
PWM0_setup(PWM_pin_is_PWM_output, \
PWM_init_lvl_low, \
PWM_0_pin_P10, \
PWM_level_normal);
PWM1_setup(PWM_pin_is_PWM_output, \
PWM_init_lvl_low, \
PWM_1_pin_P11, \
PWM_level_normal);
PWM2_setup(PWM_pin_is_PWM_output, \
PWM_init_lvl_low, \
PWM_2_pin_P12, \
PWM_level_normal);
PWM_set_PWM0_T1(0);
PWM_set_PWM0_T2(0);
PWM_set_PWM1_T1(0);
PWM_set_PWM1_T2(0);
PWM_set_PWM2_T1(0);
PWM_set_PWM2_T2(0);
PWM_start_counter;
To achieve smooth fading the duty cycle of each LED is mapped using a sine table and the duty cycles are varied according to the formula as have already seen.

However, there are phase shifts in all of these tables, meaning that the three PWM channels do not have the same duty cycle at the same time.
const unsigned int duty1_value[steps] = {0, 950, 1892, 2817, 3717, 4582, 5407, 6182, 6901, 7558, 8146,
8660, 9096, 9450, 9718, 9898, 9988, 9995, 9897, 9717, 9449, 9095,
8658, 8144, 7555, 6898, 6179, 5403, 4579, 3713, 2813, 1888 ,946};
const unsigned int duty2_value[steps] = {8658, 8144, 7555, 6898, 6179, 5403, 4579, 3713, 2813, 1888 ,946,
0, 950, 1892, 2817, 3717, 4582, 5407, 6182, 6901, 7558, 8146,
8660, 9096, 9450, 9718, 9898, 9988, 9995, 9897, 9717, 9449, 9095};
const unsigned int duty3_value[steps] = {8660, 9096, 9450, 9718, 9898, 9988, 9995, 9897, 9717, 9449, 9095,
8658, 8144, 7555, 6898, 6179, 5403, 4579, 3713, 2813, 1888 ,946,
0, 950, 1892, 2817, 3717, 4582, 5407, 6182, 6901, 7558, 8146};
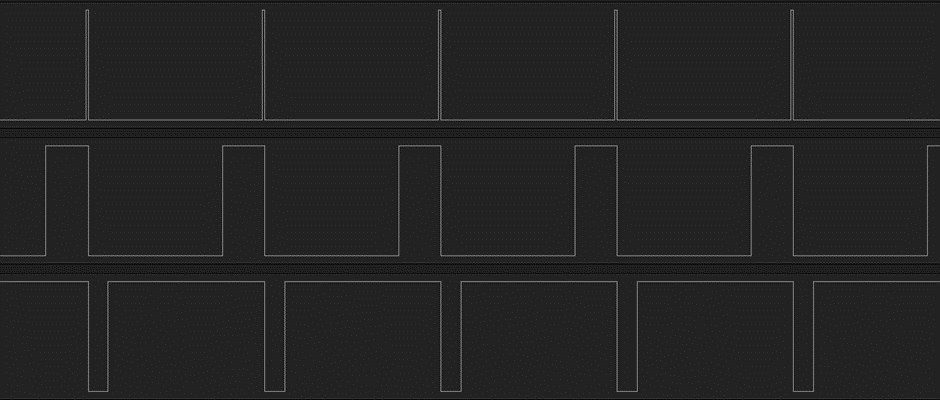
In the main, the PWM channels are driven as per their respective sine tables.
for(j = 0; j < 6; j++)
{
for(i = 0; i < steps; i++)
{
PWM_set_PWM0_T2(duty1_value[i]);
PWM_set_PWM1_T2(duty2_value[i]);
PWM_set_PWM2_T2(duty3_value[i]);
delay_ms(60);
}
}
PWM_idle();
for(j = 0; j < 6; j++)
{
for(i = 0; i < steps; i++)
{
PWM_set_PWM0_T2(duty1_value[i]);
PWM_set_PWM1_T2(duty1_value[i]);
PWM_set_PWM2_T2(duty1_value[i]);
delay_ms(60);
}
}
PWM_idle();
Note there is new function in this example unlike the last one. The PWM_idle function ensures that PWM channels are reset once it is called.
void PWM_idle(void)
{
PWM0_hold_level(PWM_HLD_L_low);
PWM1_hold_level(PWM_HLD_L_low);
PWM2_hold_level(PWM_HLD_L_low);
delay_ms(100);
PWM0_hold_level(PWM_level_normal);
PWM1_hold_level(PWM_level_normal);
PWM2_hold_level(PWM_level_normal);
delay_ms(100);
}
Demo
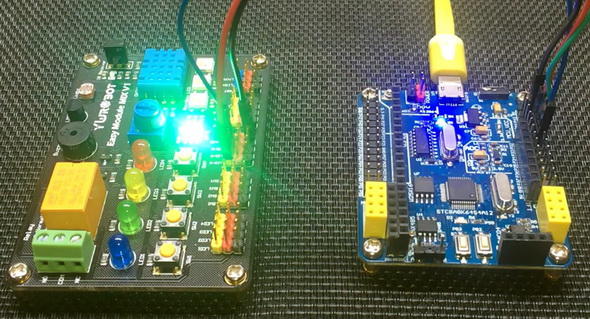
|
hello.
this is a very good effort to document all and still share with us. thank you very much.
I have one doubt . which programming tool are you using ?
Hi, I am trying to understand the STC15w408as chip, and found this site after weeks of searching for something that sets the output of the GPIO pins to a different state. I have a the 28 pin stc15w and have connected it up with a FTDI board and can write to it using PlatformIO. The thing is, the GPIO ports if just switched on or do a reset they are in the HIGH state and I am trying to make them LOW when you do a reset.
Is your BSP code doing this and for what port or GPIO pin is it setting? I could change your P52 and P55 in your SETUP to the GPIO pins on my development board but not under standing the BSP Code.
Wonder if you get this post? but any help would be gratefully received.
Hi,
How Purchase the development board. Please,give the purchase link for this Development board.
https://www.alibaba.com/product-detail/Development-board-1T-STC8A8K64S4A12-single-chip_62391507065.html
https://world.taobao.com/item/600882463994.htm
https://www.amazon.ca/STC8A8K64S4A12-Development-Controller-Module-Minimal/dp/B08D3Y3R6T
How To read and write string data using IAP into memory
void IAP_erase(unsigned int address)
{
IAP_CONTR = 0x80; //?? IAP
IAP_TPS = 12;
// IAP_CONTR = IAP_WT;
IAP_CMD = IAP_erase_command;
IAP_address(address);
IAP_trigger;
_nop_();
_nop_();
_nop_();
IAP_clear;
}
void IAP_send_string(unsigned int uc_send_addr,unsigned char *uca_send_string,unsigned int uc_number_of_bytes)
{
unsigned int buff_cntr=0;
do
{
IAP_CONTR = 0x80; //?? ISP/IAP ??
IAP_TPS = (unsigned char)(11509200 / 1000000L); //??????
IAP_CMD = IAP_write_command;
// IAP_CMD = IAP_write_command;
IAP_ADDRH = uc_send_addr / 256; //??????(??????????????)
IAP_ADDRL = uc_send_addr % 256; //??????
IAP_DATA = uca_send_string[buff_cntr]; //???? ISP_DATA,????????????
IAP_trigger;//IAP_TRIG();
_nop_();
_nop_();
_nop_();
uc_send_addr++;
// uca_send_string++;
buff_cntr++;
IAP_clear;
delay_ms(8);
}while(–uc_number_of_bytes);
}
void IAP_read_string(unsigned int uc_read_addr,unsigned char *data_read,unsigned int uc_number_of_bytes)
{
unsigned int buff_cntr=0;
do{
IAP_CONTR = 0x80; //?? ISP/IAP ??
IAP_TPS = (unsigned char)(11059200 / 1000000L); //??????
IAP_CMD = IAP_read_command;
// IAP_CMD = IAP_read_command;
IAP_ADDRH = uc_read_addr / 256; //??????(??????????????)
IAP_ADDRL = uc_read_addr % 256; //??????
IAP_trigger;//IAP_TRIG(); //?? 5AH,?? A5H ? ISP/IAP ?????,
//???????
//?? A5H ?, ISP/IAP ?????????
//CPU ?? IAP ???,?????????
_nop_();
_nop_();
_nop_();
data_read[buff_cntr] = IAP_DATA; //???????
uc_read_addr++;
// data_read++;
buff_cntr++;
IAP_clear;
delay_ms(8);
}while(–uc_number_of_bytes);
}
stores only last byte to all bytes of flash memory sector… memory sector selected is 0xF600
Hi, I am using STC MCU since 10 years. Tech support is ZERO. but they are low cost, very stable. Now I have a problem when the chip that I used is obsolete. Now start to use STC8C2K64S4-28I-LQFP32 but no stc8Cxx.h file, I am using stc8Hxx.h file which compiles but in some stage freeze, the existing firmware. With stc8hxx.h file I can compile STC8F2K64S4-28I-LQFP32 and works not bad
.
I wrote them many times for the stc8Cxx.h file never got answer. Where Can I find that file?
Thank you
Give me detail 8f2k64s281MCU read and write programmer
Give me detail 8f2k64s281reed and write programmer distal
Hi. Can you explain how to use I2C in the slave mode ?
I tried STC8G1K08A i2c in slave mode. Doesn’t work (no response). It does not enter interrupt, even on a start condition (everything according to the code in the documentation). I also tried master mode – it works.
Thanks for these tutorials. I’m getting back into STCmicro coding now, having left them alone for the past several years. Back then I only used the STC89C52RC (and C54RD) but this time I’m also using the more powerful STC15 and STC8 types. Your blogs provide a wealth of useful information.
Hello,
You have done great job with all these tutorials. I am an electronics engineer trying to learn some new stuff. I am located in Greece , Europe and I would like to purchase the development board that you are using and download some datasheets in English if possible but I cannot find them anywhere. Could you please help me?
I suggest you buy from AliExpress or similar platform that is available in your country…. You can find the English datasheet here. English documentation can be found in STC’s official websites such as this one….
Thank you very much for your help!!!
i always get excited when you release new tutorials ,you are really doing a great job i wish i could write code and develop libraries like you.
Well, this is very nice and thorough tutorial indeed, many thanks!
Unfortunately I doubt there is good any reason to learn the STC platform beyond curiosity.
The STC 8051, although pretty evolved from the original 8051 ISA, does not offer anything crucial to justify the relatively high price of these micros and development tools along with certain cumbersomeness of this ancient platform.
They simply can not compete even with the legacy Cortex M0 in any way. I am even not aware about any affordable debugger/emulator for them.
All in all, I would never recommend anybody to start learning/using any 8051 without some very good reason to do so.