Tinkering TI MSP430F5529
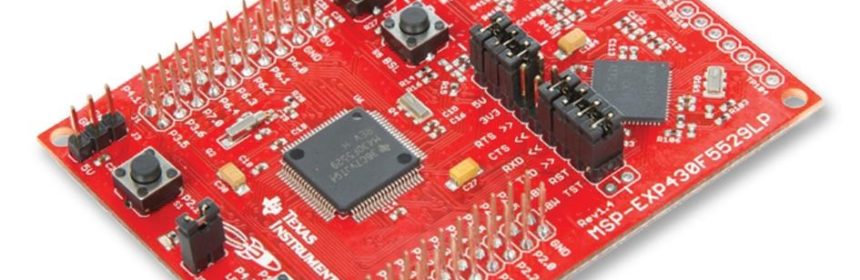
|
Watchdog Timer – WDTA
The prime task of a watchdog timer is to reset a microcontroller should there be any software irresponsiveness. MSP430F5529’s watchdog timer, WDTA can be used like a regular watchdog timer as well as an interval timer. WDTA is similar to the previously seen WDT+ but it is a bit advanced in some areas like 32-bit counter instead of 16-bit counter. Shown below is the block diagram of WDTA:
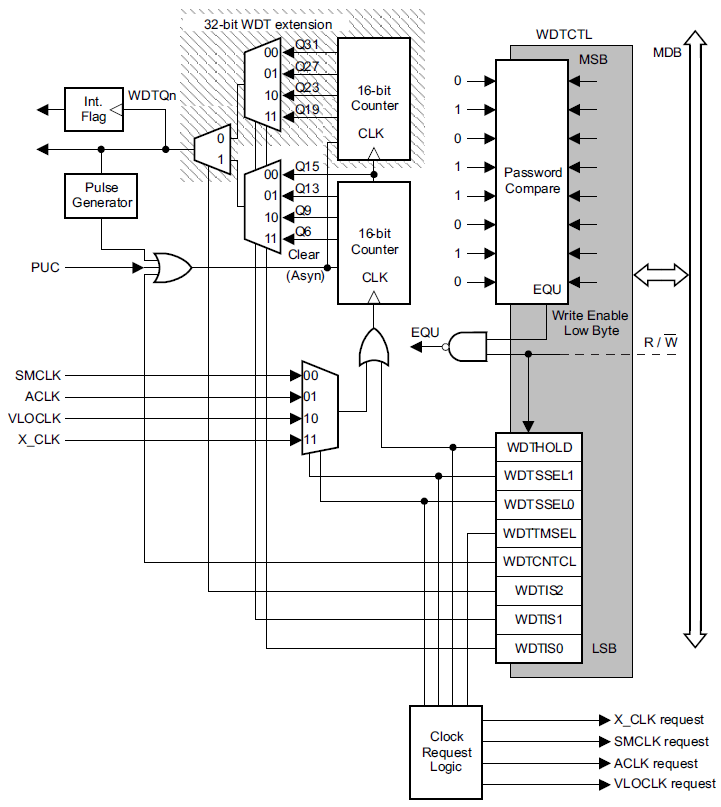
Unlike the watchdogs of many other microcontrollers, WDTA is password protected and it means that to read/write it, a special code (0x05A) needs to be set first.
Code Example
#include "driverlib.h" #include "delay.h" void clock_init(void); void GPIO_init(void); void WDTA_init(void); void main(void) { WDT_A_hold(WDT_A_BASE); clock_init(); GPIO_init(); WDTA_init(); while(1) { GPIO_toggleOutputOnPin(GPIO_PORT_P1, GPIO_PIN0); delay_ms(600); WDT_A_resetTimer(WDT_A_BASE); if(GPIO_getInputPinValue(GPIO_PORT_P1, GPIO_PIN1) == false) { GPIO_setOutputHighOnPin(GPIO_PORT_P4, GPIO_PIN7); while(1); } }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_3, UCS_XCAP_3); UCS_initClockSignal(UCS_FLLREF, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_4); UCS_initFLLSettle(MCLK_KHZ, MCLK_FLLREF_RATIO); UCS_initClockSignal(UCS_SMCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_2); UCS_initClockSignal(UCS_ACLK, UCS_XT1CLK_SELECT, UCS_CLOCK_DIVIDER_1); } void GPIO_init(void) { GPIO_setAsInputPinWithPullUpResistor(GPIO_PORT_P1, GPIO_PIN1); GPIO_setAsOutputPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setDriveStrength(GPIO_PORT_P1, GPIO_PIN0, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setDriveStrength(GPIO_PORT_P4, GPIO_PIN7, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setOutputLowOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setOutputLowOnPin(GPIO_PORT_P4, GPIO_PIN7); } void WDTA_init(void) { WDT_A_initWatchdogTimer(WDT_A_BASE, WDT_A_CLOCKSOURCE_VLOCLK, WDT_A_CLOCKDIVIDER_32K); WDT_A_start(WDT_A_BASE); WDT_A_resetTimer(WDT_A_BASE); }
Hardware Setup
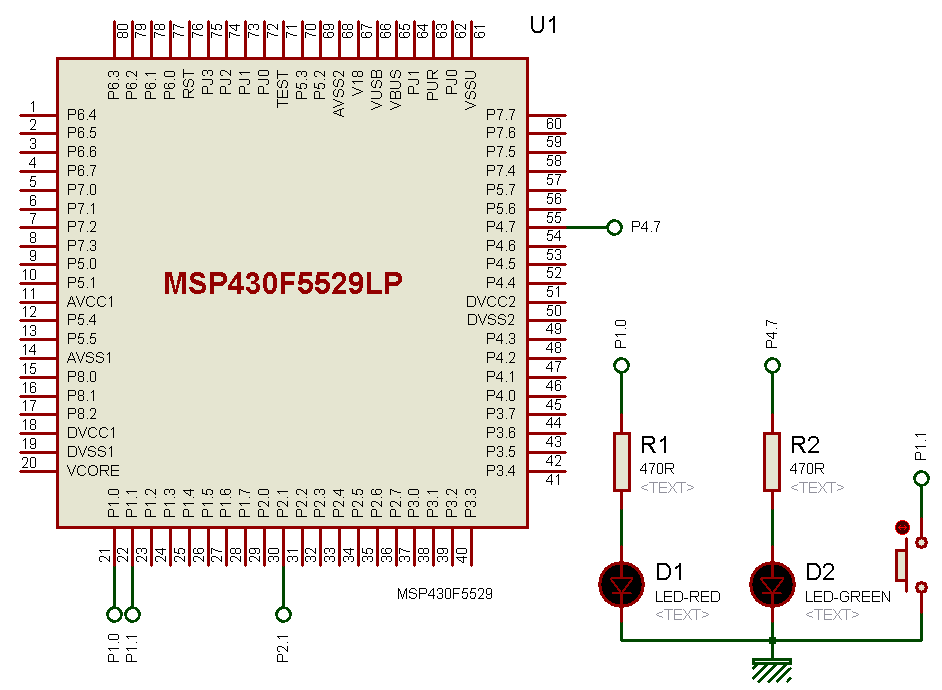
Explanation
After power-on reset, WDTA is configured in watchdog mode with an approximate initial 32 ms reset interval. Unless commanded to stop immediately, it will keep resetting MCU. This is why at the start of every program the following code is placed:
WDT_A_hold(WDT_A_BASE);
This piece of code disables WDTA until reconfigured and reused.
To setup WDTA in watchdog mode, we need to specify its source of clock signal. In this example, it is VLOCLK. We also need to decide the WDTA clock divider in order to achieve the required amount of period.
void WDTA_init(void) { WDT_A_initWatchdogTimer(WDT_A_BASE, WDT_A_CLOCKSOURCE_VLOCLK, WDT_A_CLOCKDIVIDER_32K); WDT_A_start(WDT_A_BASE); WDT_A_resetTimer(WDT_A_BASE); }
Here the time period according to the settings is about 3 seconds (32000 / 10 kHz). If WDTA is not reset within this time window, a reset will be triggered.
In the main, P1.0 LED is toggled every 600ms and WDTA is reset. Thus, WDTA counter is reset 5 times earlier than reset interval. This goes on until P1.1 push button is pressed. When the button is pressed, P4.7 LED is lit and a predefined intentional software loop is entered. Since WDTA counter is no longer reset in the loop, WDTA counter keeps ticking and a reset occurs after 3 seconds, starting everything from the beginning.
GPIO_toggleOutputOnPin(GPIO_PORT_P1, GPIO_PIN0); delay_ms(600); WDT_A_resetTimer(WDT_A_BASE); if(GPIO_getInputPinValue(GPIO_PORT_P1, GPIO_PIN1) == false) { GPIO_setOutputHighOnPin(GPIO_PORT_P4, GPIO_PIN7); while(1); }
Note that after reset, RAM data are usually not erased unless modified or reinitialized. However, in real life applications, RAM data may be corrupted because something unusual caused the reset to occur. Therefore, we can use CRC or other means to check RAM data integrity if past data are needed.
Demo
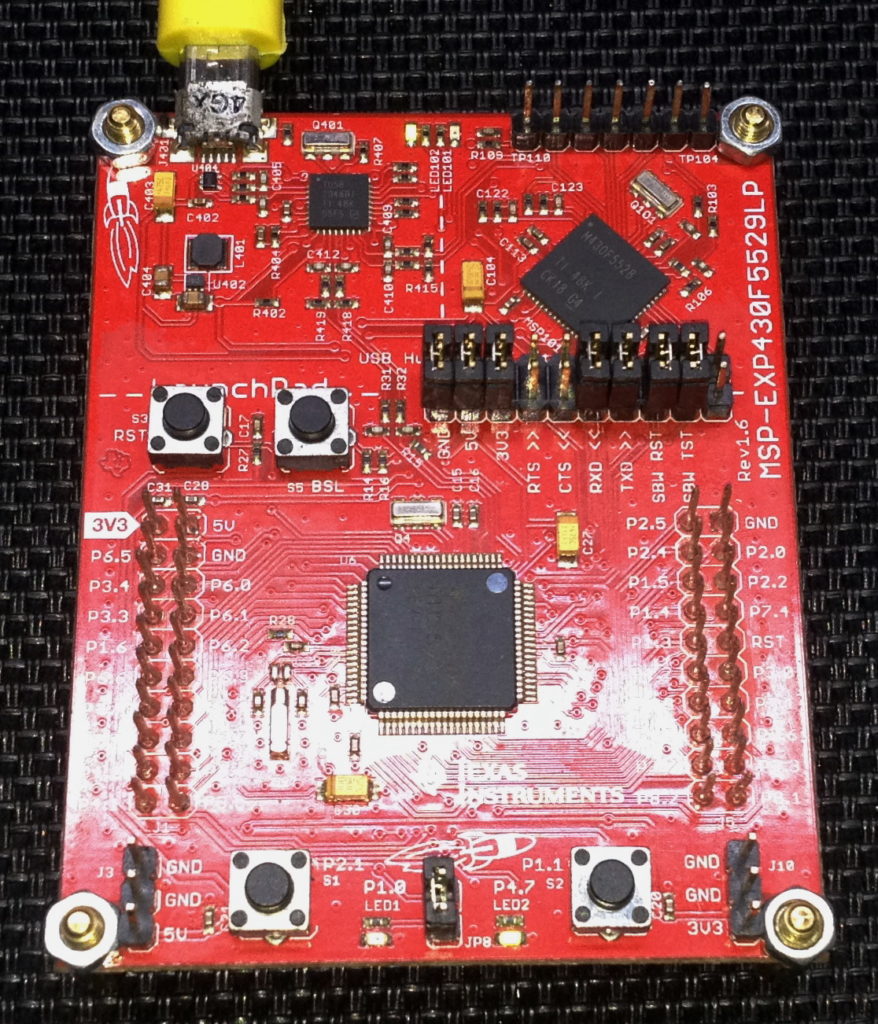
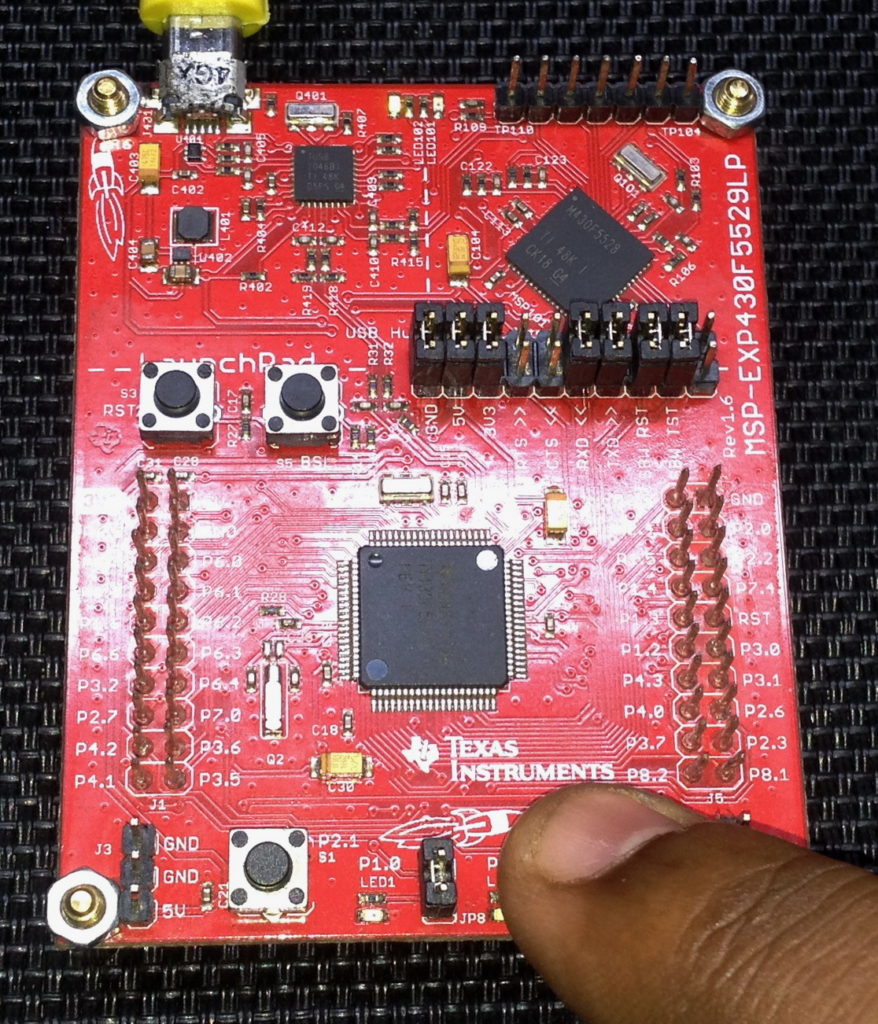
|
Hello, what software are you using for the Hardware setup images and does it support simulation for the MSP430F5529
I used Proteus VSM for drawing schematics but it doesn’t support simulation.
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True