Tinkering TI MSP430F5529
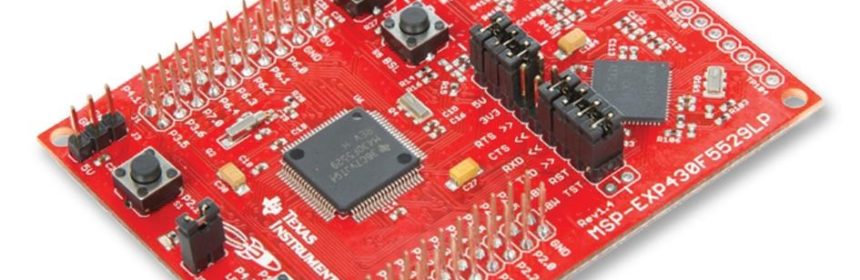
|
USCI – SPI – I2C Example 3
Two examples SPI-I2C would have been enough but went for this final third one because sometimes it is difficult for grasping I2C/SPI operations with driverlib alone. This is especially the case when people are migrating from low-end or mid-range MSP430s that have no driverlib support and this is what happened with me at the beginning. It was then I decided what if I can use the good old I2C and SPI libraries that TI supplied for their low and mid-range devices. This example demonstrates exactly that. Here both the SPI and I2C hardware of MSP430F5529 were tested against the older libraries. These libraries do not apply the use of driverlib APIs except for pin declarations. I would also like readers to check my past SPI and I2C tutorials on MSP430G2xxx series for better reference and understanding. Here’s the link: http://embedded-lab.com/blog/more-on-ti-msp430s for those tutorials.
Note that unlike MSP430G2xxx devices, MSP430F5529 doesn’t have any USI hardware and instead of it we will have to stick to USCI modules – USCI_B modules for I2C and USCI_A or USCI_B modules for SPI communications.
If still things are still messy with USCI, we can use software-based I2C/SPI libraries but those won’t perform like the hardware-based USCI ones. In terms of communication speed, software-based I2C/SPI are slow and in terms of coding, software-based solutions are resource-consuming. Thus, it is best to learn and use USCI modules.
Here in this demo, an I2C-based BH1750 light sensor is read and its readings are displayed on a SPI-based Nokia GLCD (PCD8544).
Code Example
USCI_I2C.h
#include<msp430.h> #include "driverlib.h" #define USCI_B0_I2C_port GPIO_PORT_P3 #define USCI_B0_I2C_SDA_pin GPIO_PIN0 #define USCI_B0_I2C_SCL_pin GPIO_PIN1 #define USCI_B1_I2C_port GPIO_PORT_P4 #define USCI_B1_I2C_SDA_pin GPIO_PIN1 #define USCI_B1_I2C_SCL_pin GPIO_PIN2 void I2C_USCI_B0_init(unsigned char address); void I2C_USCI_B0_set_address(unsigned char address); unsigned char I2C_USCI_B0_read_byte(unsigned char address); unsigned char I2C_USCI_B0_read_word(unsigned char address,unsigned char *value, unsigned char length); unsigned char I2C_USCI_B0_write_byte(unsigned char address, unsigned char value); void I2C_USCI_B1_init(unsigned char address); void I2C_USCI_B1_set_address(unsigned char address); unsigned char I2C_USCI_B1_read_byte(unsigned char address); unsigned char I2C_USCI_B1_read_word(unsigned char address,unsigned char *value, unsigned char length); unsigned char I2C_USCI_B1_write_byte(unsigned char address, unsigned char value);
USCI_I2C.c
#include "USCI_I2C.h" void I2C_USCI_B0_init(unsigned char address) { GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B0_I2C_port, (USCI_B0_I2C_SDA_pin | USCI_B0_I2C_SCL_pin)); UCB0CTL1 |= UCSWRST; UCB0CTL0 = (UCMST | UCMODE_3 | UCSYNC); UCB0CTL1 = (UCSSEL_2 | UCSWRST); UCB0BR0 = 50; UCB0I2CSA = address; UCB0CTL1 &= ~UCSWRST; } void I2C_USCI_B0_set_address(unsigned char address) { UCB0CTL1 |= UCSWRST; UCB0I2CSA = address; UCB0CTL1 &= ~UCSWRST; } unsigned char I2C_USCI_B0_read_byte(unsigned char address) { while(UCB0CTL1 & UCTXSTP); UCB0CTL1 |= (UCTR | UCTXSTT); while(!(UCB0IFG & UCTXIFG)); UCB0TXBUF = address; while(!(UCB0IFG & UCTXIFG)); UCB0CTL1 &= ~UCTR; UCB0CTL1 |= UCTXSTT; UCB0IFG &= ~UCTXIFG; while(UCB0CTL1 & UCTXSTT); UCB0CTL1 |= UCTXSTP; return UCB0RXBUF; } unsigned char I2C_USCI_B0_read_word(unsigned char address,unsigned char *value, unsigned char length) { unsigned char i = 0; while(UCB0CTL1 & UCTXSTP); UCB0CTL1 |= (UCTR | UCTXSTT); while(!(UCB0IFG & UCTXIFG)); UCB0IFG &= ~UCTXIFG; if(UCB0IFG & UCNACKIFG) { return UCB0STAT; } UCB0TXBUF = address; while(!(UCB0IFG & UCTXIFG)); if(UCB0IFG & UCNACKIFG) { return UCB0STAT; } UCB0CTL1 &= ~UCTR; UCB0CTL1 |= UCTXSTT; UCB0IFG &= ~UCTXIFG; while(UCB0CTL1 & UCTXSTT); do { while (!(UCB0IFG & UCRXIFG)); UCB0IFG &= ~UCTXIFG; value[i] = UCB0RXBUF; i++; }while(i < (length - 1)); while (!(UCB0IFG & UCRXIFG)); UCB0IFG &= ~UCTXIFG; UCB0CTL1 |= UCTXSTP; value[length - 1] = UCB0RXBUF; UCB0IFG &= ~UCTXIFG; return 0; } unsigned char I2C_USCI_B0_write_byte(unsigned char address, unsigned char value) { while(UCB0CTL1 & UCTXSTP); UCB0CTL1 |= (UCTR | UCTXSTT); while(!(UCB0IFG & UCTXIFG)); if(UCB0IFG & UCNACKIFG) { return UCB0STAT; } UCB0TXBUF = address; while(!(UCB0IFG & UCTXIFG)); if(UCB0IFG & UCNACKIFG) { return UCB0STAT; } UCB0TXBUF = value; while(!(UCB0IFG & UCTXIFG)); if(UCB0IFG & UCNACKIFG) { return UCB0STAT; } UCB0CTL1 |= UCTXSTP; UCB0IFG &= ~UCTXIFG; return 0; } void I2C_USCI_B1_init(unsigned char address) { GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B1_I2C_port, (USCI_B1_I2C_SDA_pin | USCI_B1_I2C_SCL_pin)); UCB1CTL1 |= UCSWRST; UCB1CTL0 = (UCMST | UCMODE_3 | UCSYNC); UCB1CTL1 = (UCSSEL_2 | UCSWRST); UCB1BR0 = 10; UCB1I2CSA = address; UCB1CTL1 &= ~UCSWRST; } void I2C_USCI_B1_set_address(unsigned char address) { UCB1CTL1 |= UCSWRST; UCB1I2CSA = address; UCB1CTL1 &= ~UCSWRST; } unsigned char I2C_USCI_B1_read_byte(unsigned char address) { while(UCB1CTL1 & UCTXSTP); UCB1CTL1 |= (UCTR | UCTXSTT); while(!(UCB1IFG & UCTXIFG)); UCB1TXBUF = address; while(!(UCB1IFG & UCTXIFG)); UCB1CTL1 &= ~UCTR; UCB1CTL1 |= UCTXSTT; UCB1IFG &= ~UCTXIFG; while(UCB1CTL1 & UCTXSTT); UCB1CTL1 |= UCTXSTP; return UCB1RXBUF; } unsigned char I2C_USCI_B1_read_word(unsigned char address,unsigned char *value, unsigned char length) { unsigned char i = 0; while(UCB1CTL1 & UCTXSTP); UCB1CTL1 |= (UCTR | UCTXSTT); while(!(UCB1IFG & UCTXIFG)); UCB1IFG &= ~UCTXIFG; if(UCB1IFG & UCNACKIFG) { return UCB1STAT; } UCB1TXBUF = address; while(!(UCB1IFG & UCTXIFG)); if(UCB1IFG & UCNACKIFG) { return UCB1STAT; } UCB1CTL1 &= ~UCTR; UCB1CTL1 |= UCTXSTT; UCB1IFG &= ~UCTXIFG; while(UCB1CTL1 & UCTXSTT); do { while (!(UCB1IFG & UCRXIFG)); UCB1IFG &= ~UCTXIFG; value[i] = UCB1RXBUF; i++; }while(i < (length - 1)); while (!(UCB1IFG & UCRXIFG)); UCB1IFG &= ~UCTXIFG; UCB1CTL1 |= UCTXSTP; value[length - 1] = UCB1RXBUF; UCB1IFG &= ~UCTXIFG; return 0; } unsigned char I2C_USCI_B1_write_byte(unsigned char address, unsigned char value) { while(UCB1CTL1 & UCTXSTP); UCB1CTL1 |= (UCTR | UCTXSTT); while(!(UCB1IFG & UCTXIFG)); if(UCB1IFG & UCNACKIFG) { return UCB1STAT; } UCB1TXBUF = address; while(!(UCB1IFG & UCTXIFG)); if(UCB1IFG & UCNACKIFG) { return UCB1STAT; } UCB1TXBUF = value; while(!(UCB1IFG & UCTXIFG)); if(UCB1IFG & UCNACKIFG) { return UCB1STAT; } UCB1CTL1 |= UCTXSTP; UCB1IFG &= ~UCTXIFG; return 0; }
BH1750.h
#include<msp430.h> "driverlib.h" #include "delay.h" #include "USCI_I2C.h" #define BH1750_addr 0x23 #define power_down 0x00 #define power_up 0x01 #define reset 0x07 #define cont_H_res_mode1 0x10 #define cont_H_res_mode2 0x11 #define cont_L_res_mode 0x13 #define one_time_H_res_mode1 0x20 #define one_time_H_res_mode2 0x21 #define one_time_L_res_mode 0x23 void BH1750_init(void); void BH1750_write(unsigned char cmd); unsigned int BH1750_read_word(void); unsigned int get_lux_value(unsigned char mode, unsigned int delay_time);
BH1750.c
#include "BH1750.h" void BH1750_init(void) { I2C_USCI_B0_init(BH1750_addr); delay_ms(10); BH1750_write(power_down); } void BH1750_write(unsigned char cmd) { I2C_USCI_B0_write_byte(BH1750_addr, cmd); } unsigned int BH1750_read_word(void) { unsigned long value = 0x0000; unsigned char bytes[2] = {0x00, 0x00}; I2C_USCI_B0_read_word(0x11, bytes, 2); value = ((bytes[1] << 8) | bytes[0]); return value; } unsigned int get_lux_value(unsigned char mode, unsigned int delay_time) { unsigned long lux_value = 0x00; unsigned char dly = 0x00; unsigned char s = 0x08; while(s) { BH1750_write(power_up); BH1750_write(mode); lux_value += BH1750_read_word(); for(dly = 0; dly >= 3; return ((unsigned int)lux_value); }
USCI_SPI.h
#include<msp430.h> #include "driverlib.h" #define USCI_A0_MOSI_port GPIO_PORT_P3 #define USCI_A0_MISO_port GPIO_PORT_P3 #define USCI_A0_CLK_port GPIO_PORT_P2 #define USCI_A0_MOSI_pin GPIO_PIN3 #define USCI_A0_MISO_pin GPIO_PIN4 #define USCI_A0_CLK_pin GPIO_PIN7 #define USCI_A1_MOSI_port GPIO_PORT_P4 #define USCI_A1_MISO_port GPIO_PORT_P4 #define USCI_A1_CLK_port GPIO_PORT_P4 #define USCI_A1_MOSI_pin GPIO_PIN4 #define USCI_A1_MISO_pin GPIO_PIN5 #define USCI_A1_CLK_pin GPIO_PIN0 #define USCI_B0_MOSI_port GPIO_PORT_P3 #define USCI_B0_MISO_port GPIO_PORT_P3 #define USCI_B0_CLK_port GPIO_PORT_P3 #define USCI_B0_MOSI_pin GPIO_PIN0 #define USCI_B0_MISO_pin GPIO_PIN1 #define USCI_B0_CLK_pin GPIO_PIN2 #define USCI_B1_MOSI_port GPIO_PORT_P4 #define USCI_B1_MISO_port GPIO_PORT_P4 #define USCI_B1_CLK_port GPIO_PORT_P4 #define USCI_B1_MOSI_pin GPIO_PIN1 #define USCI_B1_MISO_pin GPIO_PIN2 #define USCI_B1_CLK_pin GPIO_PIN3 void SPI_USCI_A0_init(void); void SPI_USCI_A0_write(unsigned char tx_data); unsigned char SPI_USCI_A0_read(void); unsigned char SPI_USCI_A0_transfer(unsigned char tx_data); void SPI_USCI_A1_init(void); void SPI_USCI_A1_write(unsigned char tx_data); unsigned char SPI_USCI_A1_read(void); unsigned char SPI_USCI_A1_transfer(unsigned char tx_data); void SPI_USCI_B0_init(void); void SPI_USCI_B0_write(unsigned char tx_data); unsigned char SPI_USCI_B0_read(void); unsigned char SPI_USCI_B0_transfer(unsigned char tx_data); void SPI_USCI_B1_init(void); void SPI_USCI_B1_write(unsigned char tx_data); unsigned char SPI_USCI_B1_read(void); unsigned char SPI_USCI_B1_transfer(unsigned char tx_data);
USCI_SPI.c
#include "USCI_SPI.h" void SPI_USCI_A0_init(void) { GPIO_setAsPeripheralModuleFunctionInputPin(USCI_A0_MISO_port, USCI_A0_MISO_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_A0_MOSI_port, USCI_A0_MOSI_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_A0_CLK_port, USCI_A0_CLK_pin); UCA0CTL1 |= UCSWRST; UCA0CTL0 = UCCKPH | UCMSB | UCMST | UCMODE_1 | UCSYNC; UCA0CTL1 = UCSSEL_2; UCA0BR0 = 4; UCA0BR1 = 0; UCA0CTL1 &= ~UCSWRST; } void SPI_USCI_A0_write(unsigned char tx_data) { while(!(UCA0IFG & UCTXIFG)); UCA0TXBUF = tx_data; while(UCA0STAT & UCBUSY); } unsigned char SPI_USCI_A0_read(void) { unsigned char rx_data = 0; while(!(UCA0IFG & UCRXIFG)); rx_data = UCA0RXBUF; while(UCA0STAT & UCBUSY); return rx_data; } unsigned char SPI_USCI_A0_transfer(unsigned char tx_data) { unsigned char rx_data = 0; while(!(UCA0IFG & UCTXIFG)); UCA0TXBUF = tx_data; while(UCA0STAT & UCBUSY); while(!(UCA0IFG & UCRXIFG)); rx_data = UCA0RXBUF; while(UCA0STAT & UCBUSY); return rx_data; } void SPI_USCI_A1_init(void) { GPIO_setAsPeripheralModuleFunctionInputPin(USCI_A1_MISO_port, USCI_A1_MISO_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_A1_MOSI_port, USCI_A1_MOSI_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_A1_CLK_port, USCI_A1_CLK_pin); UCA1CTL1 |= UCSWRST; UCA1CTL0 = UCCKPH | UCMSB | UCMST | UCMODE_1 | UCSYNC; UCA1CTL1 = UCSSEL_2; UCA1BR0 = 8; UCA1BR1 = 0; UCA1CTL1 &= ~UCSWRST; } void SPI_USCI_A1_write(unsigned char tx_data) { while(!(UCA1IFG & UCTXIFG)); UCA1TXBUF = tx_data; while(UCA1STAT & UCBUSY); } unsigned char SPI_USCI_A1_read(void) { unsigned char rx_data = 0; while(!(UCA1IFG & UCRXIFG)); rx_data = UCA1RXBUF; while(UCA1STAT & UCBUSY); return rx_data; } unsigned char SPI_USCI_A1_transfer(unsigned char tx_data) { unsigned char rx_data = 0; while(!(UCA1IFG & UCTXIFG)); UCA1TXBUF = tx_data; while(UCA1STAT & UCBUSY); while(!(UCA1IFG & UCRXIFG)); rx_data = UCA1RXBUF; while(UCA1STAT & UCBUSY); return rx_data; } void SPI_USCI_B0_init(void) { GPIO_setAsPeripheralModuleFunctionInputPin(USCI_B0_MISO_port, USCI_B0_MISO_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B0_MOSI_port, USCI_B0_MOSI_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B0_CLK_port, USCI_B0_CLK_pin); UCB0CTL1 |= UCSWRST; UCB0CTL0 = UCCKPH | UCMSB | UCMST | UCMODE_1 | UCSYNC; UCB0CTL1 = UCSSEL_2; UCB0BR0 = 8; UCB0BR1 = 0; UCB0CTL1 &= ~UCSWRST; } void SPI_USCI_B0_write(unsigned char tx_data) { while(!(UCB0IFG & UCTXIFG)); UCB0TXBUF = tx_data; while(UCB0STAT & UCBUSY); } unsigned char SPI_USCI_B0_read(void) { unsigned char rx_data = 0; while(!(UCB0IFG & UCRXIFG)); rx_data = UCB0RXBUF; while(UCB0STAT & UCBUSY); return rx_data; } unsigned char SPI_USCI_B0_transfer(unsigned char tx_data) { unsigned char rx_data = 0; while(!(UCB0IFG & UCTXIFG)); UCB0TXBUF = tx_data; while(UCB0STAT & UCBUSY); while(!(UCB0IFG & UCRXIFG)); rx_data = UCB0RXBUF; while(UCB0STAT & UCBUSY); return rx_data; } void SPI_USCI_B1_init(void) { GPIO_setAsPeripheralModuleFunctionInputPin(USCI_B1_MISO_port, USCI_B1_MISO_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B1_MOSI_port, USCI_B1_MOSI_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B1_CLK_port, USCI_B1_CLK_pin); UCB1CTL1 |= UCSWRST; UCB1CTL0 = UCCKPH | UCMSB | UCMST | UCMODE_1 | UCSYNC; UCB1CTL1 = UCSSEL_2; UCB1BR0 = 8; UCB1BR1 = 0; UCB1CTL1 &= ~UCSWRST; } void SPI_USCI_B1_write(unsigned char tx_data) { while(!(UCB1IFG & UCTXIFG)); UCB1TXBUF = tx_data; while(UCB1STAT & UCBUSY); } unsigned char SPI_USCI_B1_read(void) { unsigned char rx_data = 0; while(!(UCB1IFG & UCRXIFG)); rx_data = UCB1RXBUF; while(UCB1STAT & UCBUSY); return rx_data; } unsigned char SPI_USCI_B1_transfer(unsigned char tx_data) { unsigned char rx_data = 0; while(!(UCB1IFG & UCTXIFG)); UCB1TXBUF = tx_data; while(UCB1STAT & UCBUSY); while(!(UCB1IFG & UCRXIFG)); rx_data = UCB1RXBUF; while(UCB1STAT & UCBUSY); return rx_data; }
PCD8544.h
#include "driverlib.h" #include "delay.h" #include "USCI_SPI.h" #define RST_port GPIO_PORT_P6 #define CS_port GPIO_PORT_P6 #define DC_port GPIO_PORT_P6 #define RST_pin GPIO_PIN0 #define CS_pin GPIO_PIN1 #define DC_pin GPIO_PIN2 #define RST_pin_high() GPIO_setOutputHighOnPin(RST_port, RST_pin) #define RST_pin_low() GPIO_setOutputLowOnPin(RST_port, RST_pin) #define CS_pin_high() GPIO_setOutputHighOnPin(CS_port, CS_pin) #define CS_pin_low() GPIO_setOutputLowOnPin(CS_port, CS_pin) #define DC_pin_high() GPIO_setOutputHighOnPin(DC_port, DC_pin) #define DC_pin_low() GPIO_setOutputLowOnPin(DC_port, DC_pin) #define PCD8544_set_Y_addr 0x40 #define PCD8544_set_X_addr 0x80 #define PCD8544_set_temp 0x04 #define PCD8544_set_bias 0x10 #define PCD8544_set_VOP 0x80 #define PCD8544_power_down 0x04 #define PCD8544_entry_mode 0x02 #define PCD8544_extended_instruction 0x01 #define PCD8544_display_blank 0x00 #define PCD8544_display_normal 0x04 #define PCD8544_display_all_on 0x01 #define PCD8544_display_inverted 0x05 #define PCD8544_function_set 0x20 #define PCD8544_display_control 0x08 #define CMD 0 #define DAT 1 #define X_max 84 #define Y_max 48 #define Rows 6 #define BLACK 0 #define WHITE 1 #define PIXEL_XOR 2 #define ON 1 #define OFF 0 #define NO 0 #define YES 1 #define buffer_size 504 void PCD8544_init(void); void PCD8544_reset(void); void PCD8544_write(unsigned char type, unsigned char value); void PCD8544_set_contrast(unsigned char value); void PCD8544_set_cursor(unsigned char x_pos, unsigned char y_pos); void PCD8544_print_char(unsigned char ch, unsigned char colour); void PCD8544_print_custom_char(unsigned char *map); void PCD8544_fill(unsigned char bufr); void PCD8544_clear_buffer(unsigned char colour); void PCD8544_clear_screen(unsigned char colour); void print_image(const unsigned char *bmp); void print_char(unsigned char x_pos, unsigned char y_pos, unsigned char ch, unsigned char colour); void print_string(unsigned char x_pos, unsigned char y_pos, unsigned char *ch, unsigned char colour); void print_chr(unsigned char x_pos, unsigned char y_pos, signed int value, unsigned char colour); void print_int(unsigned char x_pos, unsigned char y_pos, signed long value, unsigned char colour); void print_decimal(unsigned char x_pos, unsigned char y_pos, unsigned int value, unsigned char points, unsigned char colour); void print_float(unsigned char x_pos, unsigned char y_pos, float value, unsigned char points, unsigned char colour); void Draw_Pixel(unsigned char x_pos, unsigned char y_pos, unsigned char colour); void Draw_Line(signed int x1, signed int y1, signed int x2, signed int y2, unsigned char colour); void Draw_Rectangle(signed int x1, signed int y1, signed int x2, signed int y2, unsigned char fill, unsigned char colour); void Draw_Circle(signed int xc, signed int yc, signed int radius, unsigned char fill, unsigned char colour);
PCD8544.c
#include "PCD8544.h" static unsigned char PCD8544_buffer[X_max][Rows]; static const unsigned char font[96][5] = { {0x00, 0x00, 0x00, 0x00, 0x00} // 20 ,{0x00, 0x00, 0x5f, 0x00, 0x00} // 21 ! ,{0x00, 0x07, 0x00, 0x07, 0x00} // 22 " ,{0x14, 0x7f, 0x14, 0x7f, 0x14} // 23 # ,{0x24, 0x2a, 0x7f, 0x2a, 0x12} // 24 $ ,{0x23, 0x13, 0x08, 0x64, 0x62} // 25 % ,{0x36, 0x49, 0x55, 0x22, 0x50} // 26 & ,{0x00, 0x05, 0x03, 0x00, 0x00} // 27 ' ,{0x00, 0x1c, 0x22, 0x41, 0x00} // 28 ( ,{0x00, 0x41, 0x22, 0x1c, 0x00} // 29 ) ,{0x14, 0x08, 0x3e, 0x08, 0x14} // 2a * ,{0x08, 0x08, 0x3e, 0x08, 0x08} // 2b + ,{0x00, 0x50, 0x30, 0x00, 0x00} // 2c , ,{0x08, 0x08, 0x08, 0x08, 0x08} // 2d - ,{0x00, 0x60, 0x60, 0x00, 0x00} // 2e . ,{0x20, 0x10, 0x08, 0x04, 0x02} // 2f / ,{0x3e, 0x51, 0x49, 0x45, 0x3e} // 30 0 ,{0x00, 0x42, 0x7f, 0x40, 0x00} // 31 1 ,{0x42, 0x61, 0x51, 0x49, 0x46} // 32 2 ,{0x21, 0x41, 0x45, 0x4b, 0x31} // 33 3 ,{0x18, 0x14, 0x12, 0x7f, 0x10} // 34 4 ,{0x27, 0x45, 0x45, 0x45, 0x39} // 35 5 ,{0x3c, 0x4a, 0x49, 0x49, 0x30} // 36 6 ,{0x01, 0x71, 0x09, 0x05, 0x03} // 37 7 ,{0x36, 0x49, 0x49, 0x49, 0x36} // 38 8 ,{0x06, 0x49, 0x49, 0x29, 0x1e} // 39 9 ,{0x00, 0x36, 0x36, 0x00, 0x00} // 3a : ,{0x00, 0x56, 0x36, 0x00, 0x00} // 3b ; ,{0x08, 0x14, 0x22, 0x41, 0x00} // 3c ,{0x02, 0x01, 0x51, 0x09, 0x06} // 3f ? ,{0x32, 0x49, 0x79, 0x41, 0x3e} // 40 @ ,{0x7e, 0x11, 0x11, 0x11, 0x7e} // 41 A ,{0x7f, 0x49, 0x49, 0x49, 0x36} // 42 B ,{0x3e, 0x41, 0x41, 0x41, 0x22} // 43 C ,{0x7f, 0x41, 0x41, 0x22, 0x1c} // 44 D ,{0x7f, 0x49, 0x49, 0x49, 0x41} // 45 E ,{0x7f, 0x09, 0x09, 0x09, 0x01} // 46 F ,{0x3e, 0x41, 0x49, 0x49, 0x7a} // 47 G ,{0x7f, 0x08, 0x08, 0x08, 0x7f} // 48 H ,{0x00, 0x41, 0x7f, 0x41, 0x00} // 49 I ,{0x20, 0x40, 0x41, 0x3f, 0x01} // 4a J ,{0x7f, 0x08, 0x14, 0x22, 0x41} // 4b K ,{0x7f, 0x40, 0x40, 0x40, 0x40} // 4c L ,{0x7f, 0x02, 0x0c, 0x02, 0x7f} // 4d M ,{0x7f, 0x04, 0x08, 0x10, 0x7f} // 4e N ,{0x3e, 0x41, 0x41, 0x41, 0x3e} // 4f O ,{0x7f, 0x09, 0x09, 0x09, 0x06} // 50 P ,{0x3e, 0x41, 0x51, 0x21, 0x5e} // 51 Q ,{0x7f, 0x09, 0x19, 0x29, 0x46} // 52 R ,{0x46, 0x49, 0x49, 0x49, 0x31} // 53 S ,{0x01, 0x01, 0x7f, 0x01, 0x01} // 54 T ,{0x3f, 0x40, 0x40, 0x40, 0x3f} // 55 U ,{0x1f, 0x20, 0x40, 0x20, 0x1f} // 56 V ,{0x3f, 0x40, 0x38, 0x40, 0x3f} // 57 W ,{0x63, 0x14, 0x08, 0x14, 0x63} // 58 X ,{0x07, 0x08, 0x70, 0x08, 0x07} // 59 Y ,{0x61, 0x51, 0x49, 0x45, 0x43} // 5a Z ,{0x00, 0x7f, 0x41, 0x41, 0x00} // 5b [ ,{0x02, 0x04, 0x08, 0x10, 0x20} // 5c ? ,{0x00, 0x41, 0x41, 0x7f, 0x00} // 5d ] ,{0x04, 0x02, 0x01, 0x02, 0x04} // 5e ^ ,{0x40, 0x40, 0x40, 0x40, 0x40} // 5f _ ,{0x00, 0x01, 0x02, 0x04, 0x00} // 60 ` ,{0x20, 0x54, 0x54, 0x54, 0x78} // 61 a ,{0x7f, 0x48, 0x44, 0x44, 0x38} // 62 b ,{0x38, 0x44, 0x44, 0x44, 0x20} // 63 c ,{0x38, 0x44, 0x44, 0x48, 0x7f} // 64 d ,{0x38, 0x54, 0x54, 0x54, 0x18} // 65 e ,{0x08, 0x7e, 0x09, 0x01, 0x02} // 66 f ,{0x0c, 0x52, 0x52, 0x52, 0x3e} // 67 g ,{0x7f, 0x08, 0x04, 0x04, 0x78} // 68 h ,{0x00, 0x44, 0x7d, 0x40, 0x00} // 69 i ,{0x20, 0x40, 0x44, 0x3d, 0x00} // 6a j ,{0x7f, 0x10, 0x28, 0x44, 0x00} // 6b k ,{0x00, 0x41, 0x7f, 0x40, 0x00} // 6c l ,{0x7c, 0x04, 0x18, 0x04, 0x78} // 6d m ,{0x7c, 0x08, 0x04, 0x04, 0x78} // 6e n ,{0x38, 0x44, 0x44, 0x44, 0x38} // 6f o ,{0x7c, 0x14, 0x14, 0x14, 0x08} // 70 p ,{0x08, 0x14, 0x14, 0x18, 0x7c} // 71 q ,{0x7c, 0x08, 0x04, 0x04, 0x08} // 72 r ,{0x48, 0x54, 0x54, 0x54, 0x20} // 73 s ,{0x04, 0x3f, 0x44, 0x40, 0x20} // 74 t ,{0x3c, 0x40, 0x40, 0x20, 0x7c} // 75 u ,{0x1c, 0x20, 0x40, 0x20, 0x1c} // 76 v ,{0x3c, 0x40, 0x30, 0x40, 0x3c} // 77 w ,{0x44, 0x28, 0x10, 0x28, 0x44} // 78 x ,{0x0c, 0x50, 0x50, 0x50, 0x3c} // 79 y ,{0x44, 0x64, 0x54, 0x4c, 0x44} // 7a z ,{0x00, 0x08, 0x36, 0x41, 0x00} // 7b { ,{0x00, 0x00, 0x7f, 0x00, 0x00} // 7c | ,{0x00, 0x41, 0x36, 0x08, 0x00} // 7d } ,{0x10, 0x08, 0x08, 0x10, 0x08} // 7e ? ,{0x78, 0x46, 0x41, 0x46, 0x78} // 7f ? }; void PCD8544_init() { GPIO_setAsOutputPin(RST_port, RST_pin); GPIO_setDriveStrength(RST_port, RST_pin, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(CS_port, CS_pin); GPIO_setDriveStrength(CS_port, CS_pin, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(DC_port, DC_pin); GPIO_setDriveStrength(DC_port, DC_pin, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); SPI_USCI_A0_init(); PCD8544_reset(); PCD8544_write(CMD, (PCD8544_extended_instruction | PCD8544_function_set)); PCD8544_write(CMD, (PCD8544_set_bias | 0x02)); PCD8544_set_contrast(0x39); PCD8544_write(CMD, PCD8544_set_temp); PCD8544_write(CMD, (PCD8544_display_normal | PCD8544_display_control)); PCD8544_write(CMD, PCD8544_function_set); PCD8544_write(CMD, PCD8544_display_all_on); PCD8544_write(CMD, PCD8544_display_normal); PCD8544_clear_buffer(OFF); PCD8544_clear_screen(ON); } void PCD8544_reset(void) { RST_pin_low(); delay_us(100); RST_pin_high(); } void PCD8544_write(unsigned char type, unsigned char value) { switch(type) { case 1: { DC_pin_high(); break; } default: { DC_pin_low(); break; } } CS_pin_low(); SPI_USCI_A0_write(value); CS_pin_high(); } void PCD8544_set_contrast(unsigned char value) { if(value >= 0x7F) { value = 0x7F; } PCD8544_write(CMD, (PCD8544_extended_instruction | PCD8544_function_set)); PCD8544_write(CMD, (PCD8544_set_VOP | value)); PCD8544_write(CMD, PCD8544_function_set); } void PCD8544_set_cursor(unsigned char x_pos, unsigned char y_pos) { PCD8544_write(CMD, (PCD8544_set_X_addr | x_pos)); PCD8544_write(CMD, (PCD8544_set_Y_addr | y_pos)); } void PCD8544_print_char(unsigned char ch, unsigned char colour) { unsigned char s = 0; unsigned char chr = 0; for(s = 0; s <= 4; s++) { chr = font[(ch - 0x20)][s]; if(colour == BLACK) { chr = ~chr; } PCD8544_write(DAT, chr); } } void PCD8544_print_custom_char(unsigned char *map) { unsigned char s = 0; for(s = 0; s <= 4; s++) { PCD8544_write(DAT, *map++); } } void PCD8544_fill(unsigned char bufr) { unsigned int s = 0; PCD8544_set_cursor(0, 0); for(s = 0; s -1) { while(y_pos > -1) { PCD8544_buffer[x_pos][y_pos] = colour; y_pos--; } x_pos--; } } void PCD8544_clear_screen(unsigned char colour) { unsigned char x_pos = 0; unsigned char y_pos = 0; for(y_pos = 0; y_pos < Rows; y_pos++) { for(x_pos = 0; x_pos < X_max; x_pos++) { print_string(x_pos, y_pos, " ", colour); } } } void print_image(const unsigned char *bmp) { unsigned int s = 0; PCD8544_set_cursor(0, 0); for(s = 0; s = 0x20) && (*ch <= 0x7F)); } void print_chr(unsigned char x_pos, unsigned char y_pos, signed int value, unsigned char colour) { unsigned char ch = 0x00; if(value 99) && (value 9) && (value = 0) && (value <= 9)) { ch = (value % 10); PCD8544_set_cursor((x_pos + 6), y_pos); PCD8544_print_char((48 + ch), colour); PCD8544_set_cursor((x_pos + 12), y_pos); PCD8544_print_char(0x20, colour); PCD8544_set_cursor((x_pos + 18), y_pos); PCD8544_print_char(0x20, colour); } } void print_int(unsigned char x_pos, unsigned char y_pos, signed long value, unsigned char colour) { unsigned char ch = 0x00; if(value 9999) { ch = (value / 10000); PCD8544_set_cursor((x_pos + 6), y_pos); PCD8544_print_char((48 + ch), colour); ch = ((value % 10000)/ 1000); PCD8544_set_cursor((x_pos + 12), y_pos); PCD8544_print_char((48 + ch), colour); ch = ((value % 1000) / 100); PCD8544_set_cursor((x_pos + 18), y_pos); PCD8544_print_char((48 + ch), colour); ch = ((value % 100) / 10); PCD8544_set_cursor((x_pos + 24), y_pos); PCD8544_print_char((48 + ch), colour); ch = (value % 10); PCD8544_set_cursor((x_pos + 30), y_pos); PCD8544_print_char((48 + ch), colour); } else if((value > 999) && (value 99) && (value 9) && (value 1) { ch = ((value % 1000) / 100); PCD8544_set_cursor((x_pos + 12), y_pos); PCD8544_print_char((48 + ch), colour); if(points > 2) { ch = ((value % 100) / 10); PCD8544_set_cursor((x_pos + 18), y_pos); PCD8544_print_char((48 + ch), colour); if(points > 3) { ch = (value % 10); PCD8544_set_cursor((x_pos + 24), y_pos); PCD8544_print_char((48 + ch), colour);; } } } } void print_float(unsigned char x_pos, unsigned char y_pos, float value, unsigned char points, unsigned char colour) { signed long tmp = 0x00; tmp = ((signed long)value); print_int(x_pos, y_pos, tmp, colour); tmp = ((value - tmp) * 10000); if(tmp = 9999) && (value = 999) && (value = 99) && (value = 9) && (value < 99)) { print_decimal((x_pos + 18), y_pos, tmp, points, colour); } else if(value < 9) { print_decimal((x_pos + 12), y_pos, tmp, points, colour); if((value) = X_max) || (y_pos >= Y_max)) { return; } row = (y_pos >> 3); value = PCD8544_buffer[x_pos][row]; if(colour == BLACK) { value |= (1 << (y_pos % 8)); } else if(colour == WHITE) { value &= (~(1 << (y_pos % 8))); } else if(colour == PIXEL_XOR) { value ^= (1 << (y_pos % 8)); } PCD8544_buffer[x_pos][row] = value; PCD8544_set_cursor(x_pos, row); PCD8544_write(DAT, value); } void Draw_Line(signed int x1, signed int y1, signed int x2, signed int y2, unsigned char colour) { signed int dx = 0x0000; signed int dy = 0x0000; signed int stepx = 0x0000; signed int stepy = 0x0000; signed int fraction = 0x0000; dy = (y2 - y1); dx = (x2 - x1); if (dy < 0) { dy = -dy; stepy = -1; } else { stepy = 1; } if (dx < 0) { dx = -dx; stepx = -1; } else { stepx = 1; } dx <<= 0x01; dy < dy) { fraction = (dy - (dx >> 1)); while (x1 != x2) { if (fraction >= 0) { y1 += stepy; fraction -= dx; } x1 += stepx; fraction += dy; Draw_Pixel(x1, y1, colour); } } else { fraction = (dx - (dy >> 1)); while (y1 != y2) { if (fraction >= 0) { x1 += stepx; fraction -= dy; } y1 += stepy; fraction += dx; Draw_Pixel(x1, y1, colour); } } } void Draw_Rectangle(signed int x1, signed int y1, signed int x2, signed int y2, unsigned char fill, unsigned char colour) { unsigned char i = 0x00; unsigned char xmin = 0x00; unsigned char xmax = 0x00; unsigned char ymin = 0x00; unsigned char ymax = 0x00; if(fill != NO) { if(x1 < x2) { xmin = x1; xmax = x2; } else { xmin = x2; xmax = x1; } if(y1 < y2) { ymin = y1; ymax = y2; } else { ymin = y2; ymax = y1; } for(; xmin <= xmax; ++xmin) { for(i = ymin; i <= ymax; ++i) { Draw_Pixel(xmin, i, colour); } } } else { Draw_Line(x1, y1, x2, y1, colour); Draw_Line(x1, y2, x2, y2, colour); Draw_Line(x1, y1, x1, y2, colour); Draw_Line(x2, y1, x2, y2, colour); } } void Draw_Circle(signed int xc, signed int yc, signed int radius, unsigned char fill, unsigned char colour) { signed int a = 0x0000; signed int b = 0x0000; signed int p = 0x0000; b = radius; p = (1 - b); do { if(fill != NO) { Draw_Line((xc - a), (yc + b), (xc + a), (yc + b), colour); Draw_Line((xc - a), (yc - b), (xc + a), (yc - b), colour); Draw_Line((xc - b), (yc + a), (xc + b), (yc + a), colour); Draw_Line((xc - b), (yc - a), (xc + b), (yc - a), colour); } else { Draw_Pixel((xc + a), (yc + b), colour); Draw_Pixel((xc + b), (yc + a), colour); Draw_Pixel((xc - a), (yc + b), colour); Draw_Pixel((xc - b), (yc + a), colour); Draw_Pixel((xc + b), (yc - a), colour); Draw_Pixel((xc + a), (yc - b), colour); Draw_Pixel((xc - a), (yc - b), colour); Draw_Pixel((xc - b), (yc - a), colour); } if(p < 0) { p += (0x03 + (0x02 * a++)); } else { p += (0x05 + (0x02 * ((a++) - (b--)))); } }while(a <= b); }
main.c
#include<msp430.h> #include "driverlib.h" #include "delay.h" #include "USCI_I2C.h" #include "USCI_SPI.h" #include "PCD8544.h" #include "BH1750.h" void clock_init(void); void main(void) { unsigned int LX = 0x0000; unsigned int tmp = 0x0000; WDT_A_hold(WDT_A_BASE); clock_init(); PCD8544_init(); BH1750_init(); print_string(1, 3, "Lux:", ON); while(1) { tmp = get_lux_value(cont_H_res_mode1, 20); if(tmp > 10) { LX = tmp; } else { LX = get_lux_value(cont_H_res_mode1, 140); } print_int(35, 3, LX, ON); delay_ms(200); }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_3, UCS_XCAP_3); UCS_initClockSignal(UCS_FLLREF, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_4); UCS_initFLLSettle(MCLK_KHZ, MCLK_FLLREF_RATIO); UCS_initClockSignal(UCS_SMCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_4); UCS_initClockSignal(UCS_ACLK, UCS_XT1CLK_SELECT, UCS_CLOCK_DIVIDER_1); }
Hardware Setup
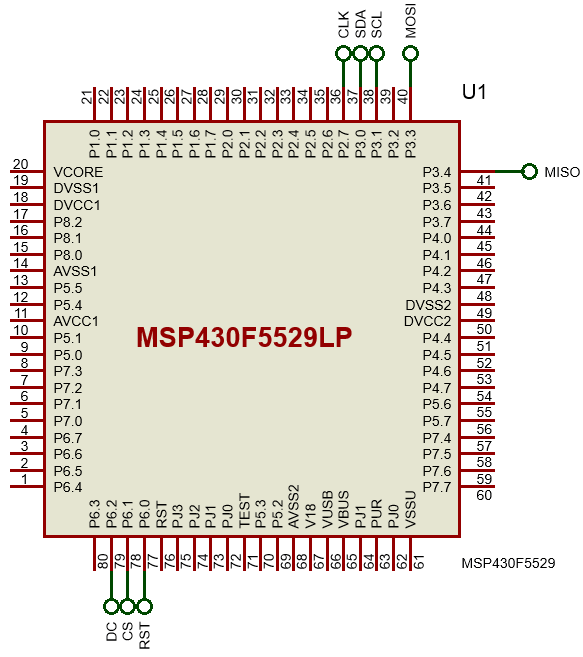
Explanation
The USCI libraries for SPI and I2C here are mostly coded at raw level and without much driverlib help. These are based on my past tutorials. I actually copied from those tutorial examples and made some minor changes. These libraries are already proven and so there is nothing new here. Basically, this USCI example is mere a proof-of-concept of the fact that we can use USCI without driverlib.
We start by declaring I/O pins as per device’s pin mapping. Let’s see the I2C library first.
#include<msp430.h> #include "driverlib.h" #define USCI_B0_I2C_port GPIO_PORT_P3 #define USCI_B0_I2C_SDA_pin GPIO_PIN0 #define USCI_B0_I2C_SCL_pin GPIO_PIN1 #define USCI_B1_I2C_port GPIO_PORT_P4 #define USCI_B1_I2C_SDA_pin GPIO_PIN1 #define USCI_B1_I2C_SCL_pin GPIO_PIN2
GPIOs to be used need to set for alternative roles.
GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_B0_I2C_port, (USCI_B0_I2C_SDA_pin | USCI_B0_I2C_SCL_pin));
The rest of the code is same as like the ones in the HW_I2C files of my past tutorial.
The demo here uses USCI B0 I2C module to operate a BH1750 light sensor as shown below:
void BH1750_write(unsigned char cmd) { I2C_USCI_B0_write_byte(BH1750_addr, cmd); } unsigned int BH1750_read_word(void) { unsigned long value = 0x0000; unsigned char bytes[2] = {0x00, 0x00}; I2C_USCI_B0_read_word(0x11, bytes, 2); value = ((bytes[1] << 8) | bytes[0]); return value; }
The same goes for the USCI SPI library.
#include<msp430.h> #include "driverlib.h" #define USCI_A0_MOSI_port GPIO_PORT_P3 #define USCI_A0_MISO_port GPIO_PORT_P3 #define USCI_A0_CLK_port GPIO_PORT_P2 #define USCI_A0_MOSI_pin GPIO_PIN3 #define USCI_A0_MISO_pin GPIO_PIN4 #define USCI_A0_CLK_pin GPIO_PIN7 #define USCI_A1_MOSI_port GPIO_PORT_P4 #define USCI_A1_MISO_port GPIO_PORT_P4 #define USCI_A1_CLK_port GPIO_PORT_P4 #define USCI_A1_MOSI_pin GPIO_PIN4 #define USCI_A1_MISO_pin GPIO_PIN5 #define USCI_A1_CLK_pin GPIO_PIN0 #define USCI_B0_MOSI_port GPIO_PORT_P3 #define USCI_B0_MISO_port GPIO_PORT_P3 #define USCI_B0_CLK_port GPIO_PORT_P3 #define USCI_B0_MOSI_pin GPIO_PIN0 #define USCI_B0_MISO_pin GPIO_PIN1 #define USCI_B0_CLK_pin GPIO_PIN2 #define USCI_B1_MOSI_port GPIO_PORT_P4 #define USCI_B1_MISO_port GPIO_PORT_P4 #define USCI_B1_CLK_port GPIO_PORT_P4 #define USCI_B1_MOSI_pin GPIO_PIN1 #define USCI_B1_MISO_pin GPIO_PIN2 #define USCI_B1_CLK_pin GPIO_PIN3
SPI has separate input and output pins and so their declaration need care.
GPIO_setAsPeripheralModuleFunctionInputPin(USCI_A0_MISO_port, USCI_A0_MISO_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_A0_MOSI_port, USCI_A0_MOSI_pin); GPIO_setAsPeripheralModuleFunctionOutputPin(USCI_A0_CLK_port, USCI_A0_CLK_pin);
Many SPI devices need additional control pins and those can be declared as ordinary GPIOs. These pins can be any pins of our choice. In this case, our Nokia GLCD has a reset, chip select and data-command pin apart from SPI communication pins.
#define RST_port GPIO_PORT_P6 #define CS_port GPIO_PORT_P6 #define DC_port GPIO_PORT_P6 #define RST_pin GPIO_PIN0 #define CS_pin GPIO_PIN1 #define DC_pin GPIO_PIN2 #define RST_pin_high() GPIO_setOutputHighOnPin(RST_port, RST_pin) #define RST_pin_low() GPIO_setOutputLowOnPin(RST_port, RST_pin) #define CS_pin_high() GPIO_setOutputHighOnPin(CS_port, CS_pin) #define CS_pin_low() GPIO_setOutputLowOnPin(CS_port, CS_pin) #define DC_pin_high() GPIO_setOutputHighOnPin(DC_port, DC_pin) #define DC_pin_low() GPIO_setOutputLowOnPin(DC_port, DC_pin) .... GPIO_setAsOutputPin(RST_port, RST_pin); GPIO_setDriveStrength(RST_port, RST_pin, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(CS_port, CS_pin); GPIO_setDriveStrength(CS_port, CS_pin, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(DC_port, DC_pin); GPIO_setDriveStrength(DC_port, DC_pin, GPIO_FULL_OUTPUT_DRIVE_STRENGTH);
The code below shows how we used the USCI_A0 in SPI mode to operate the Nokia GLCD.
void PCD8544_write(unsigned char type, unsigned char value) { switch(type) { case 1: { DC_pin_high(); break; } default: { DC_pin_low(); break; } } CS_pin_low(); SPI_USCI_A0_write(value); CS_pin_high(); }
Writing the display is same as the TFT write example. There is no read back from the GLCD.
Demo
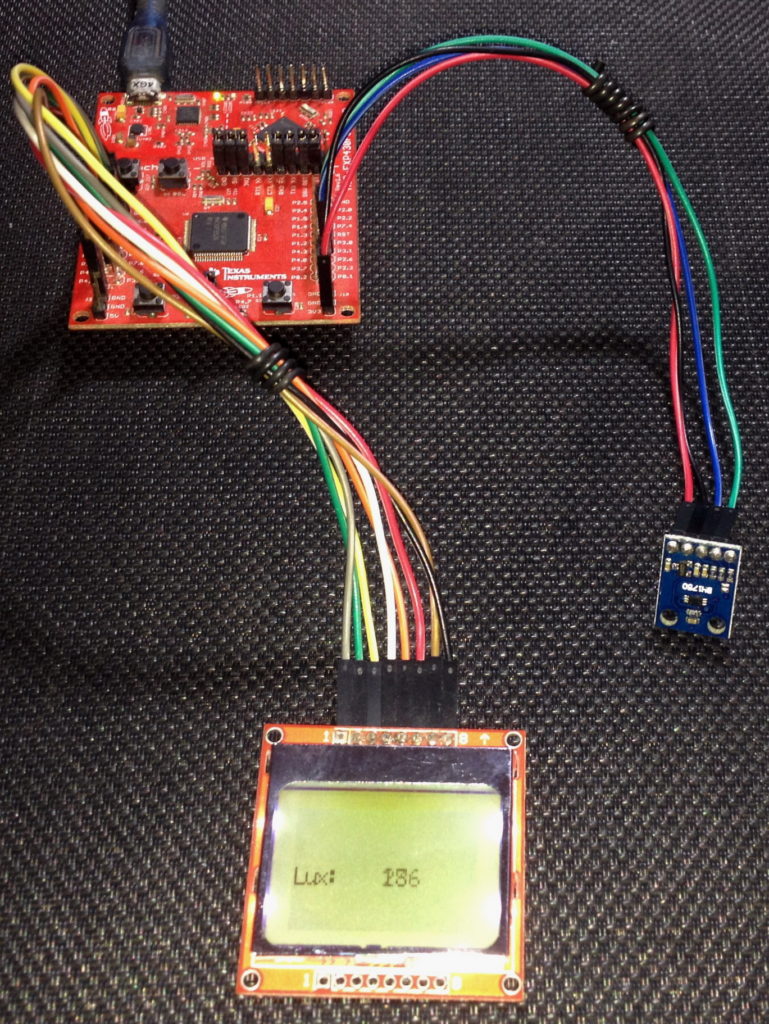
|
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True