Tinkering TI MSP430F5529
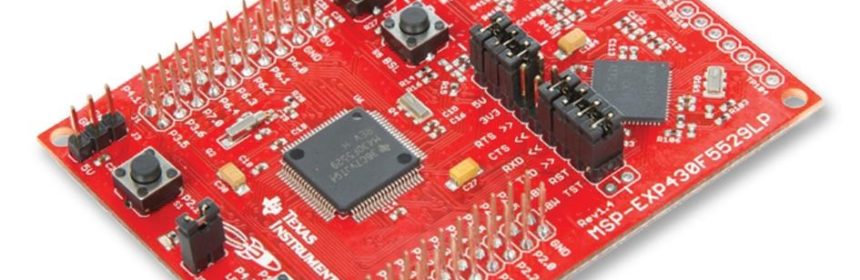
|
One-Wire Communication with DS18B20
Dallas DS18B20 is another one-wire device but as said earlier, it is different from DHT11 or other one-wire devices. DS18B20 is a high-resolution digital temperature sensor. This sensor also uses time-slotting mechanism to send and receive data from a host micro.
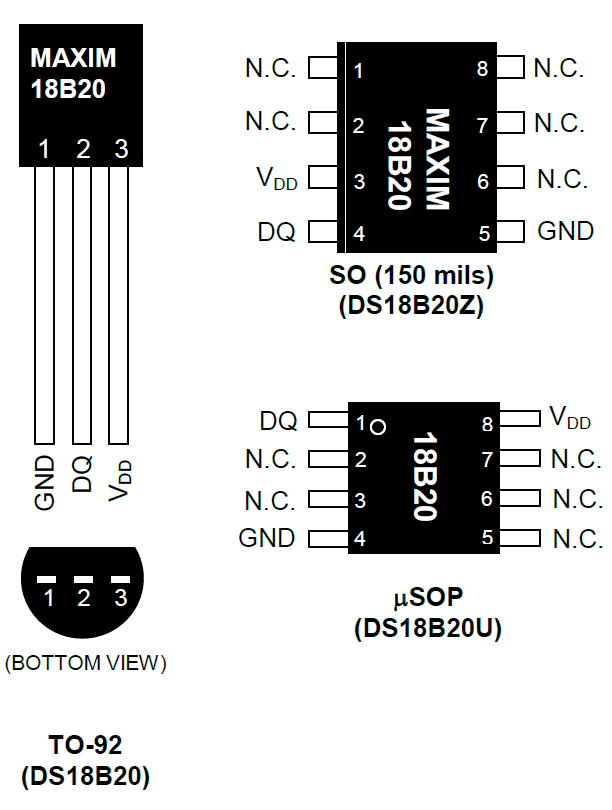
One advantage of time-slotting mechanism is the fact that no special hardware and therefore no dedicated pin of a host microcontroller is needed. Any physical pin can be used and only software delays along with polling methodology are what required. It is also possible to use internal timers instead of wasteful software delays.
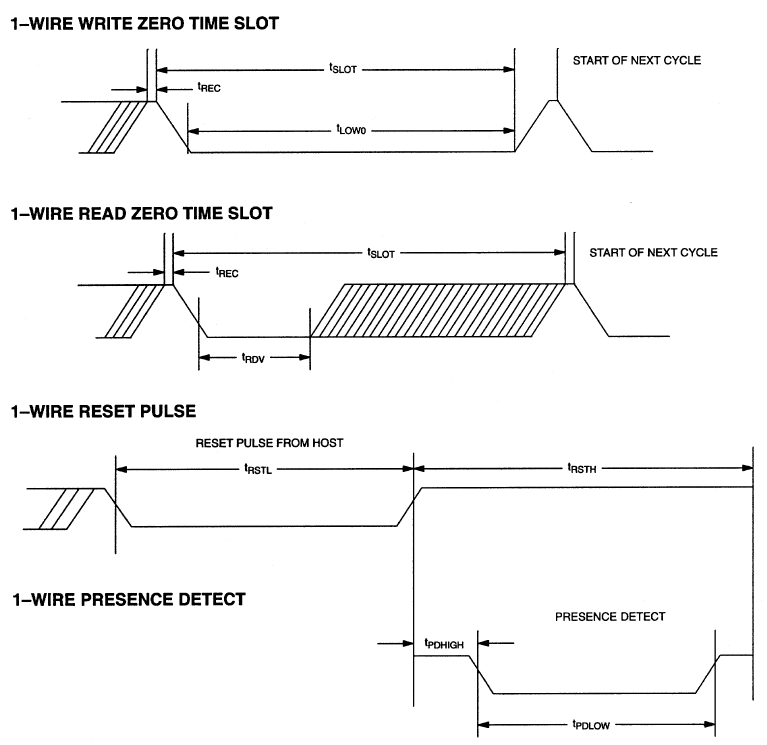
Time-slotting technique is also used in infrared remote controllers.
Code Example
one_wire.h
#include "driverlib.h" #include "delay.h" #define DS18B20_PORT GPIO_PORT_P3 #define DS18B20_PIN GPIO_PIN7 #define DS18B20_OUTPUT() GPIO_setAsOutputPin(DS18B20_PORT, DS18B20_PIN) #define DS18B20_INPUT() GPIO_setAsInputPin(DS18B20_PORT, DS18B20_PIN) #define DS18B20_IN() GPIO_getInputPinValue(DS18B20_PORT, DS18B20_PIN) #define DS18B20_OUT_HIGH() GPIO_setOutputHighOnPin(DS18B20_PORT, DS18B20_PIN) #define DS18B20_OUT_LOW() GPIO_setOutputLowOnPin(DS18B20_PORT, DS18B20_PIN) #define TRUE 1 #define FALSE 0 unsigned char onewire_reset(void) ; void onewire_write_bit(unsigned char bit_value); unsigned char onewire_read_bit(void); void onewire_write(unsigned char value); unsigned char onewire_read(void);
one_wire.c
#include "one_wire.h" unsigned char onewire_reset(void) { unsigned char res = FALSE; DS18B20_OUTPUT(); DS18B20_OUT_LOW(); delay_us(480); DS18B20_OUT_HIGH(); delay_us(60); DS18B20_INPUT(); res = DS18B20_IN(); delay_us(480); return res; } void onewire_write_bit(unsigned char bit_value) { DS18B20_OUTPUT(); DS18B20_OUT_LOW(); if(bit_value) { delay_us(104); DS18B20_OUT_HIGH(); } } unsigned char onewire_read_bit(void) { DS18B20_OUTPUT(); DS18B20_OUT_LOW(); DS18B20_OUT_HIGH(); delay_us(15); DS18B20_INPUT(); return(DS18B20_IN()); } void onewire_write(unsigned char value) { unsigned char s = 0; DS18B20_OUTPUT(); while(s < 8) { if((value & (1 << s))) { DS18B20_OUT_LOW(); _delay_cycles(1); DS18B20_OUT_HIGH(); delay_us(60); } else { DS18B20_OUT_LOW(); delay_us(60); DS18B20_OUT_HIGH(); _delay_cycles(1); } s++; } } unsigned char onewire_read(void) { unsigned char s = 0x00; unsigned char value = 0x00; while(s < 8) { DS18B20_OUTPUT(); DS18B20_OUT_LOW(); _delay_cycles(1); DS18B20_OUT_HIGH(); DS18B20_INPUT(); if(DS18B20_IN()) { value |= (1 << s); } delay_us(60); s++; } return value; }
DS18B20.h
#include "one_wire.h" #define convert_T 0x44 #define read_scratchpad 0xBE #define write_scratchpad 0x4E #define copy_scratchpad 0x48 #define recall_E2 0xB8 #define read_power_supply 0xB4 #define skip_ROM 0xCC #define resolution 12 void DS18B20_init(void); float DS18B20_get_temperature(void);
DS18B20.c
#include "DS18B20.h" void DS18B20_init(void) { onewire_reset(); delay_ms(100); } float DS18B20_get_temperature(void) { unsigned char msb = 0x00; unsigned char lsb = 0x00; register float temp = 0.0; onewire_reset(); onewire_write(skip_ROM); onewire_write(convert_T); switch(resolution) { case 12: { delay_ms(750); break; } case 11: { delay_ms(375); break; } case 10: { delay_ms(188); break; } case 9: { delay_ms(94); break; } } onewire_reset(); onewire_write(skip_ROM); onewire_write(read_scratchpad); lsb = onewire_read(); msb = onewire_read(); temp = msb; temp *= 256.0; temp += lsb; switch(resolution) { case 12: { temp *= 0.0625; break; } case 11: { temp *= 0.125; break; } case 10: { temp *= 0.25; break; } case 9: { temp *= 0.5; break; } } delay_ms(40); return (temp); }
main.c
#include "driverlib.h" #include "delay.h" #include "one_wire.h" #include "DS18B20.h" #include "lcd.h" #include "lcd_print.h" void clock_init(void); void main(void) { float t = 0.0; WDT_A_hold(WDT_A_BASE); clock_init(); DS18B20_init(); LCD_init(); load_custom_symbol(); LCD_goto(1, 0); LCD_putstr("MSP430 DS18B20"); LCD_goto(0, 1); LCD_putstr("T/ C"); print_symbol(2, 1, 0); while(1) { t = DS18B20_get_temperature(); print_F(9, 1, t, 3); delay_ms(1000); }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_0, UCS_XCAP_3); UCS_initClockSignal(UCS_MCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_SMCLK, UCS_REFOCLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_ACLK, UCS_XT1CLK_SELECT, UCS_CLOCK_DIVIDER_1); }
Hardware Setup
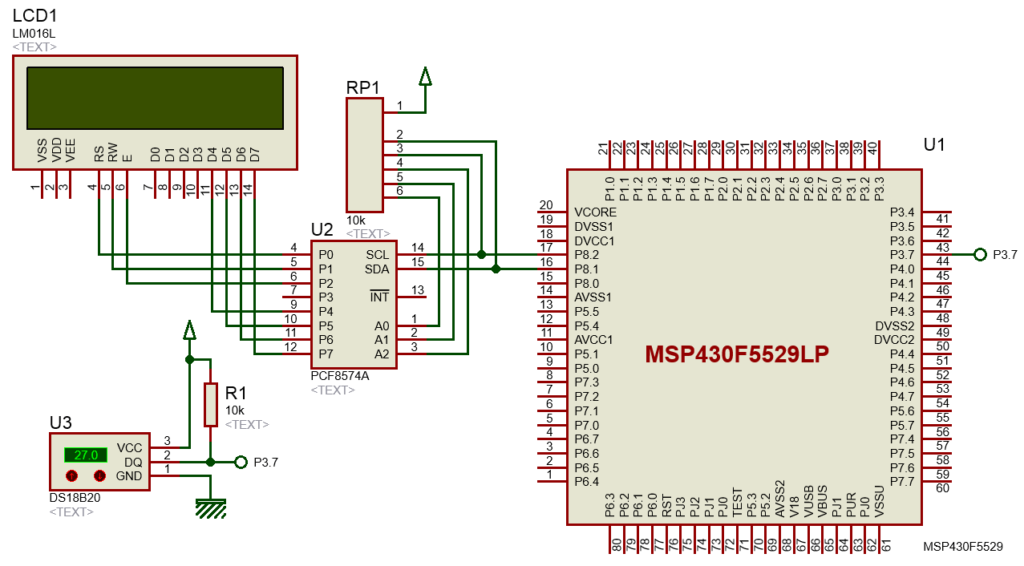
Explanation
One wire communication is detailed in these application notes from Maxim:
These notes are all that are needed for implementing the one wire communication interface for DS18B20. Please go through these notes for details. The codes are self-explanatory and are implemented from the code examples in these app notes.
Demo
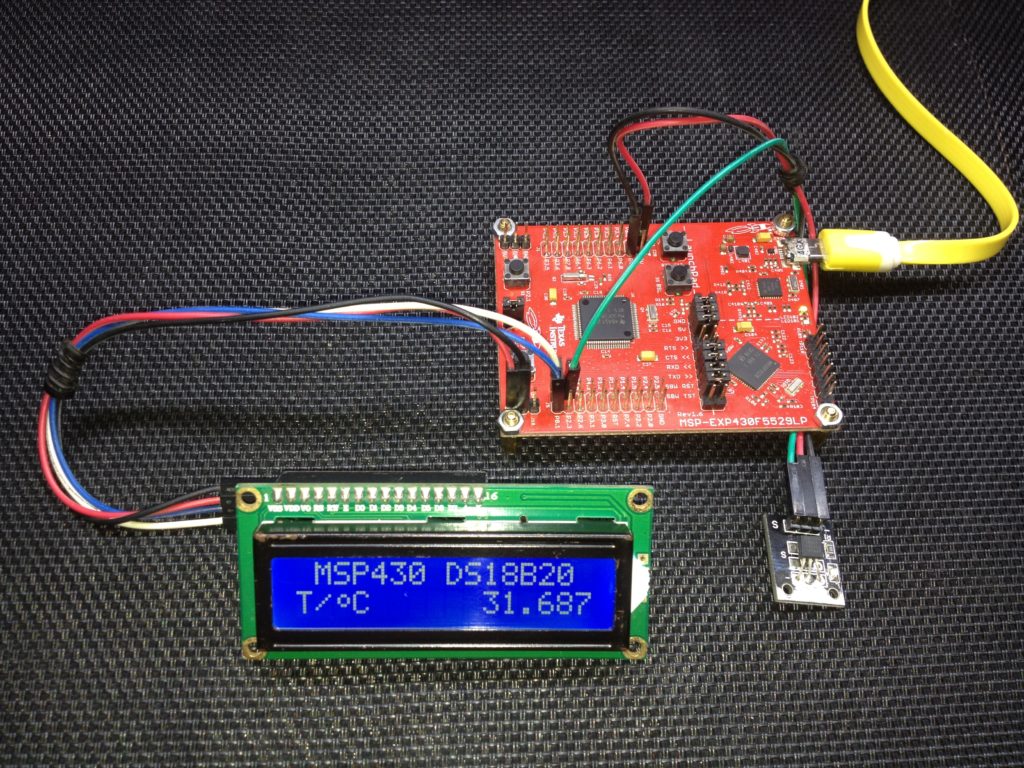
|
Hello, what software are you using for the Hardware setup images and does it support simulation for the MSP430F5529
I used Proteus VSM for drawing schematics but it doesn’t support simulation.
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True