Tinkering TI MSP430F5529
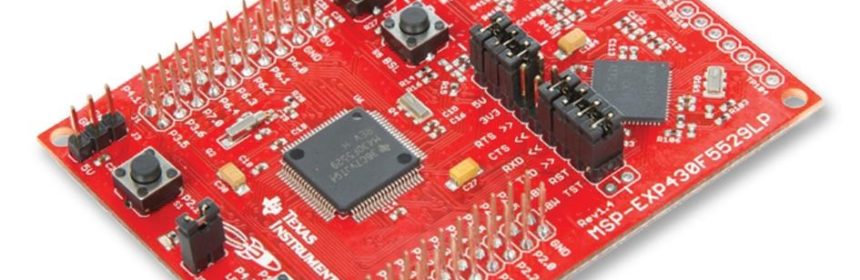
|
Digital Input-Output – DIO
Digital Input-Output (DIO) a.k.a General-Purpose Input-Output (GPIO) coding is the basic and simplest requirement for any microcontroller. This is one area where a microcontroller (MCU) differs from a microprocessor (MPU).
MSP430F5529LP Launchpad comes with 40-pin dual-in-line headers. Of these 40 pins, 35 pin headers are connected to DIO pins. There seven DIO ports but they are not evenly divided, i.e. not all ports are 8-bit wide as one would typically expect. Most of the DIOs have more than one functionality, i.e. timer, ADC, communication, etc. DIO pins can be individually programmed as either inputs or outputs. There are independent input-output data registers. In input mode, DIOs can be pulled up or pulled down using internal pull resistors just like other MSP430s. To take care of Electromagnetic Interference (EMI) issues, output drive strength can be altered in output mode, adding incredible robustness. Additionally, pins can operate at high frequency (25MHz) conditions.
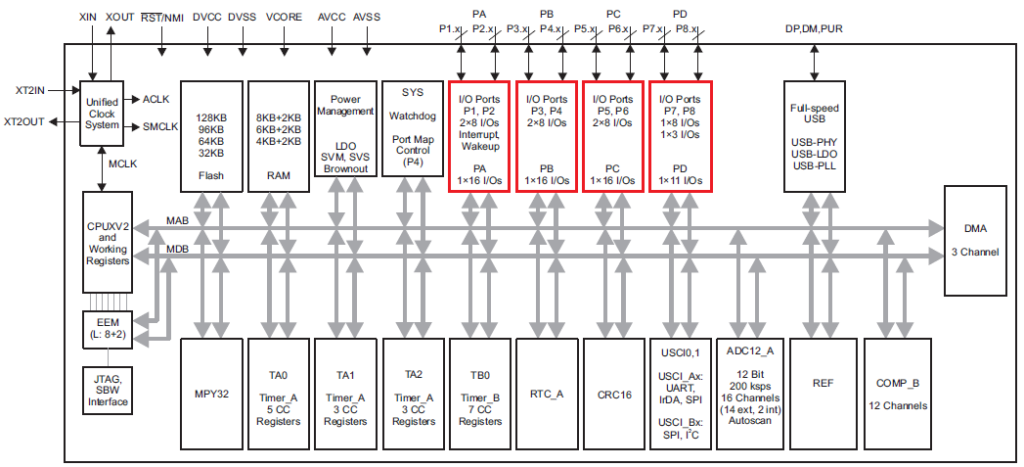
Note in the diagram above that ports are grouped as PA, PB, PC and PD. Ports are usually 8-bit wide but after adjacently grouping them as such, they become 16-bit wide. PA port pins additionally have interrupt capability unlike other ports. PA port consists of P1 and P2, PB port consists of P3 and P4 and so forth. Port grouping is helpful when we need to drive parallel port interfaces like TFT displays.
It is advised not to exceed output drive current or input voltage ranges/polarity. Best practices are to avoid driving loads directly via output pins and using buffer ICs or isolation for input pins. We must check that whether we are not overloading the microcontroller pins any how because this may have undesired consequences. Try to keep MCU’s total current consumption as low as possible. Any device is more stable at low power and optimum temperature conditions than otherwise. Remember MSP430s are low-power device.
Code Example
#include "driverlib.h" void GPIO_init(void); void main(void) { WDT_A_hold(WDT_A_BASE); GPIO_init(); while(1) { if(GPIO_getInputPinValue(GPIO_PORT_P1, GPIO_PIN1) == 0) { GPIO_setOutputHighOnPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setOutputLowOnPin(GPIO_PORT_P1, GPIO_PIN0); } if(GPIO_getInputPinValue(GPIO_PORT_P2, GPIO_PIN1) == 0) { GPIO_setOutputHighOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setOutputLowOnPin(GPIO_PORT_P4, GPIO_PIN7); } }; } void GPIO_init(void) { GPIO_setAsInputPinWithPullUpResistor(GPIO_PORT_P1, GPIO_PIN1); GPIO_setAsInputPinWithPullUpResistor(GPIO_PORT_P2, GPIO_PIN1); GPIO_setAsOutputPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setDriveStrength(GPIO_PORT_P1, GPIO_PIN0, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setDriveStrength(GPIO_PORT_P4, GPIO_PIN7, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); }
Hardware Setup
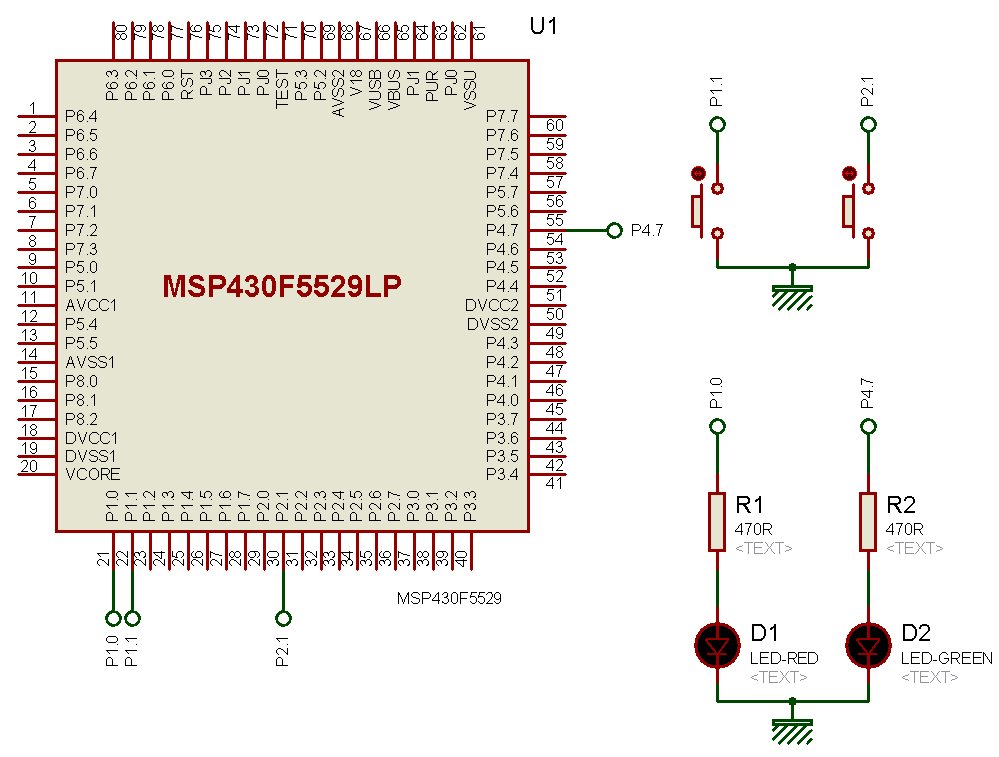
Explanation
The demo here alternatively turns on/off the pair of on-board LEDs with on-board button presses. Since this is the first example, I did not configure clocks and so the default clock settings are used. There is nothing dependent on timing.
Inputs are configured as inputs with internal pull-up resistor. This is so because the on-board buttons are directly connected the GPIO pins without any pull resistor.
GPIO_setAsInputPinWithPullUpResistor(GPIO_PORT_P1, GPIO_PIN1);
Outputs are configured full output drive output pins.
GPIO_setAsOutputPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setDriveStrength(GPIO_PORT_P4, GPIO_PIN7, GPIO_FULL_OUTPUT_DRIVE_STRENGTH);
In the main loop, the LED pins are toggle alternatively with button presses.
if(GPIO_getInputPinValue(GPIO_PORT_P1, GPIO_PIN1) == 0) { GPIO_setOutputHighOnPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setOutputLowOnPin(GPIO_PORT_P1, GPIO_PIN0); } if(GPIO_getInputPinValue(GPIO_PORT_P2, GPIO_PIN1) == 0) { GPIO_setOutputHighOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setOutputLowOnPin(GPIO_PORT_P4, GPIO_PIN7); }
Demo
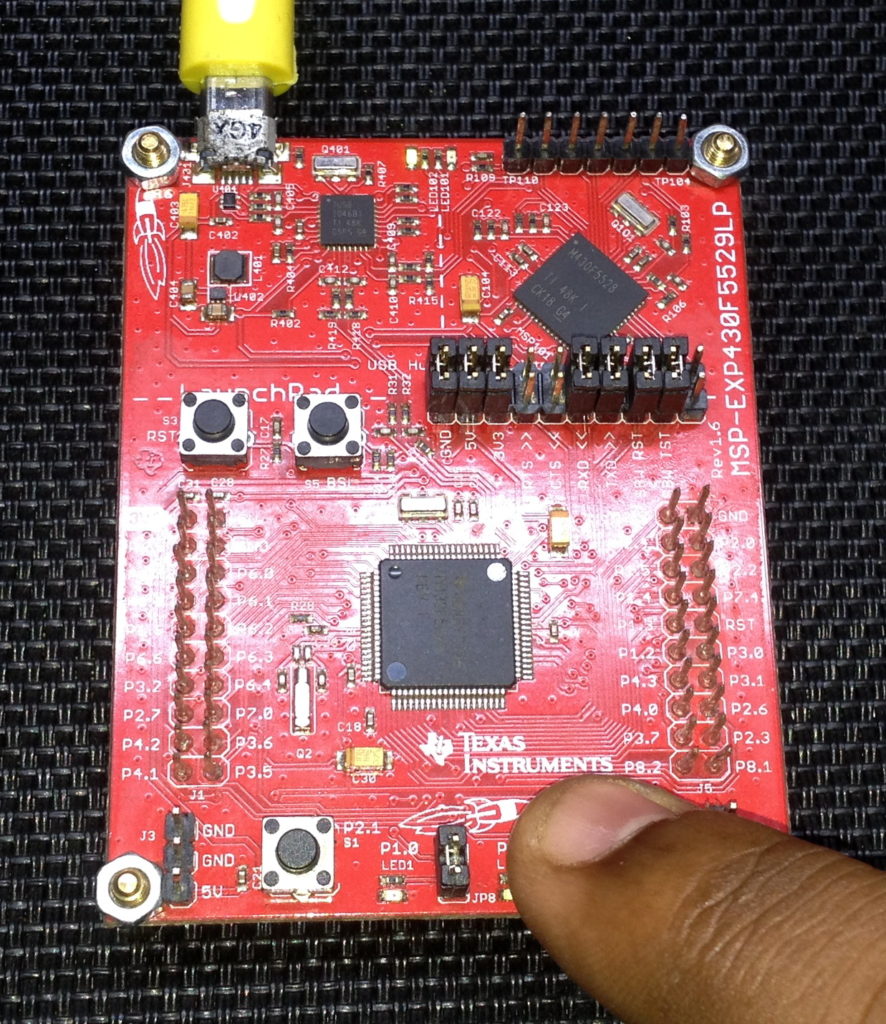
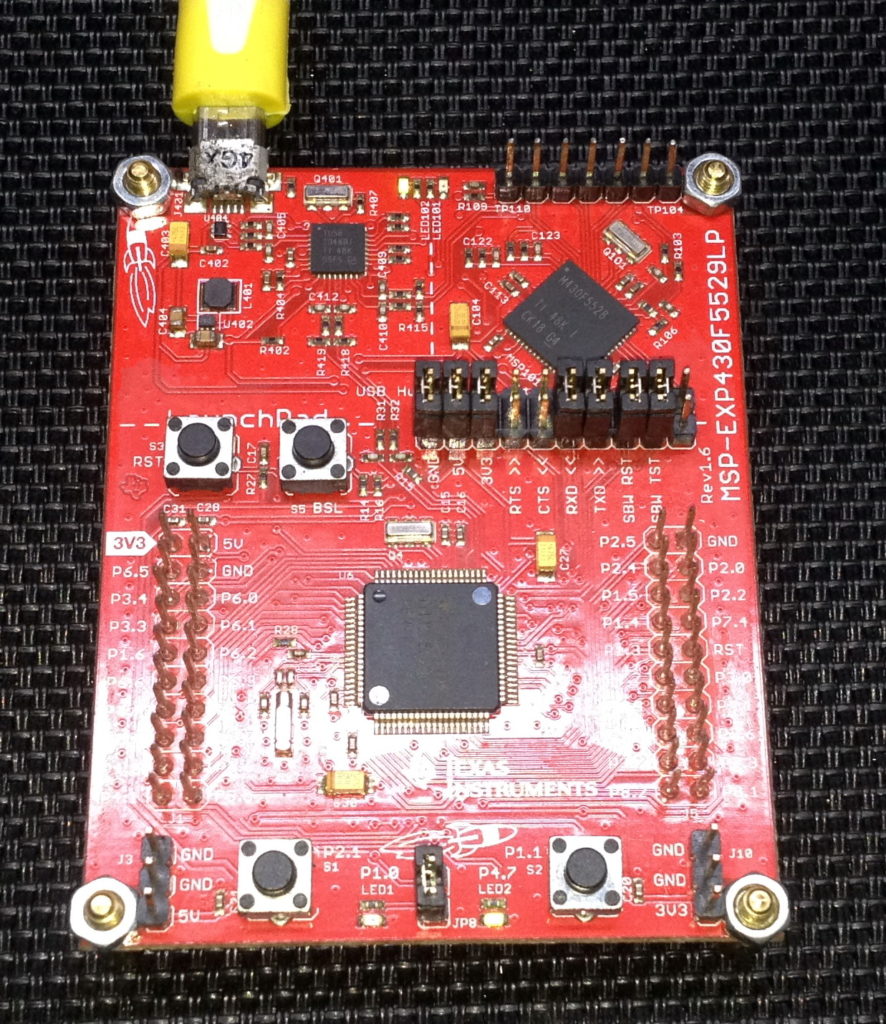
|
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True