Tinkering TI MSP430F5529
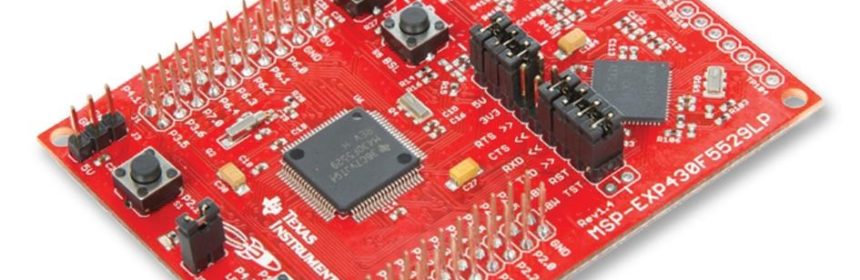
|
One-Wire Communication with DHT11
DHT11 relative humidity & temperature sensor can be interfaced with a microcontroller via one wire. However, one-wire communication is not standard format of communication like UART, I2C and SPI. Every device has its own protocol that differs a lot with others. However, the basic trick in one-wire protocol is time-slotting mechanism. Ones and zeros are represented by pulses of different pulse widths or duty cycle.
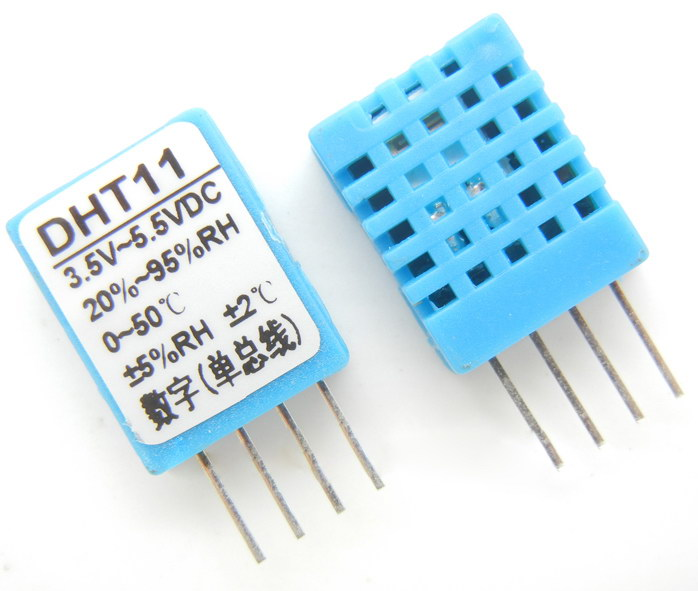
DHT11 can be used to measure temperatures nominally from 0°C to 50°C and relative humidity from 20% to 95%. Though it is not very precise, it can be used for general uses.
A big advantage of this sensor is its ability to operate at 3.3V although it is recommended to apply supply voltages between 3.5V – 5.5V.
Code Example
DHT11.h
#include "driverlib.h" #include "delay.h" #define HIGH 1 #define LOW 0 #define DHT11_PORT GPIO_PORT_P3 #define DHT11_pin GPIO_PIN7 #define DHT11_DIR_OUT() GPIO_setAsOutputPin(DHT11_PORT, DHT11_pin) #define DHT11_DIR_IN() GPIO_setAsInputPin(DHT11_PORT, DHT11_pin) #define DHT11_pin_HIGH() GPIO_setOutputHighOnPin(DHT11_PORT, DHT11_pin) #define DHT11_pin_LOW() GPIO_setOutputLowOnPin(DHT11_PORT, DHT11_pin) #define DHT11_pin_IN() GPIO_getInputPinValue(DHT11_PORT, DHT11_pin) void DHT11_init(void); unsigned char get_byte(void); unsigned char get_data(void);
DHT11.c
#include "DHT11.h" unsigned char values[0x05]; void DHT11_init(void) { DHT11_DIR_IN(); delay_ms(1000); } unsigned char get_byte(void) { unsigned char s = 0; unsigned char value = 0; DHT11_DIR_IN(); for(s = 0; s < 8; s++) { value <<= 1; while(DHT11_pin_IN() == LOW); delay_us(30); if(DHT11_pin_IN() == HIGH) { value |= 1; } while(DHT11_pin_IN() == HIGH); } return value; } unsigned char get_data(void) { short chk = 0; unsigned char s = 0; unsigned char check_sum = 0; DHT11_DIR_OUT(); DHT11_pin_HIGH(); DHT11_pin_LOW(); delay_ms(18); DHT11_pin_HIGH(); delay_us(26); DHT11_DIR_IN(); chk = DHT11_pin_IN(); if(chk) { return 1; } delay_us(80); chk = DHT11_pin_IN(); if(!chk) { return 2; } delay_us(80); for(s = 0; s <= 4; s += 1) { values[s] = get_byte(); } DHT11_DIR_OUT(); DHT11_pin_HIGH(); DHT11_DIR_IN(); for(s = 0; s < 4; s += 1) { check_sum += values[s]; } if(check_sum != values[4]) { return 3; } else { return 0; } }
main.c
#include "driverlib.h" #include "delay.h" #include "DHT11.h" #include "lcd.h" #include "lcd_print.h" extern unsigned char values[0x05]; void clock_init(void); void main(void) { unsigned char state = 0; WDT_A_hold(WDT_A_BASE); clock_init(); LCD_init(); load_custom_symbol(); DHT11_init(); while(1) { state = get_data(); switch(state) { case 1: { } case 2: { LCD_clear_home(); LCD_putstr("No Sensor Found!"); break; } case 3: { LCD_clear_home(); LCD_putstr("Checksum Error!"); break; } default: { LCD_goto(0, 0); LCD_putstr("R.H/ %: "); print_C(13, 0, values[0]); LCD_goto(0, 1); LCD_putstr("Tmp/"); print_symbol(4, 1, 0); LCD_goto(5, 1); LCD_putstr("C:"); if((values[2] & 0x80) == 1) { LCD_goto(13, 1); LCD_putstr("-"); } else { LCD_goto(13, 1); LCD_putstr(" "); } print_C(13, 1, values[2]); break; } } delay_ms(1000); }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_0, UCS_XCAP_3); UCS_initClockSignal(UCS_MCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_SMCLK, UCS_REFOCLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_ACLK, UCS_XT1CLK_SELECT, UCS_CLOCK_DIVIDER_1); }
Hardware Setup
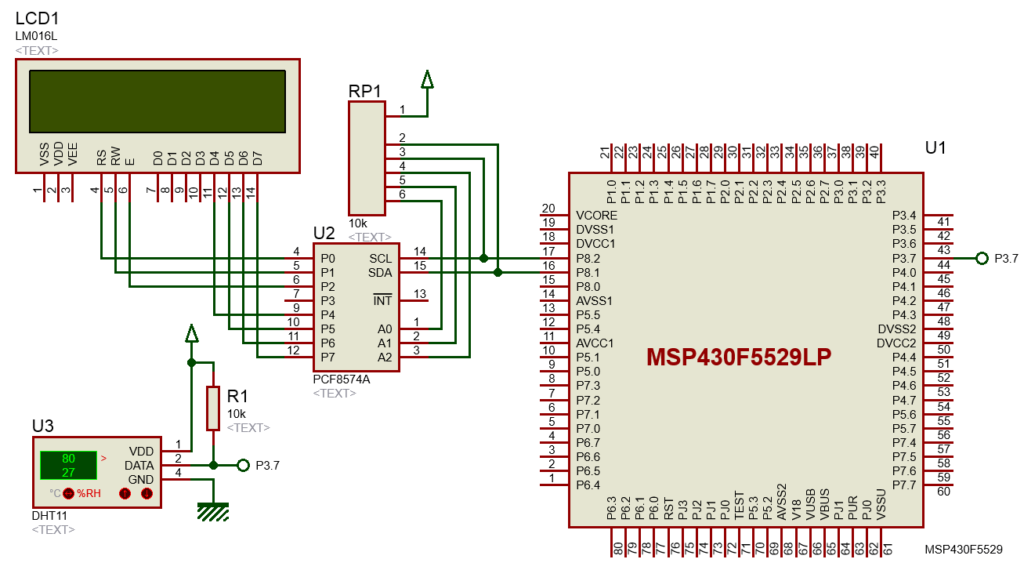
Explanation
One-wire sensors and devices use time-slotting mechanism to communicate with host micro. Time-slotting and communication methodology differ from device-to-device and have no standards like I2C, SPI, UART, etc. Despite this fact, communicating with one-wire devices is not very difficult. All we have to do is to generate timed pulse or read pulse transitions.
Shown below is the timing diagram for DHT11 relative humidity & temperature sensor. We have to keep the one-wire data line floating unless there is any communication. A floating pin is basically an externally pulled-up input pin unless used otherwise. The pull-up is achieved using an external resistor connected between the host micro’s pin and power supply’s positive line.
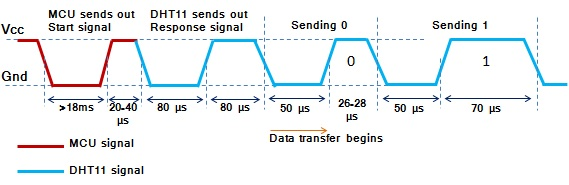
A host micro has to pull-down the floating communication low for about 18ms and then keep it high for about 30µs to command DHT11 to send out its temperature and humidity readings. This is done as follows in the code below.
DHT11_pin_HIGH(); DHT11_pin_LOW(); delay_ms(18); DHT11_pin_HIGH(); delay_us(26);
It is very straight forward. DHT11 upon receiving these logic-level transitions starts to serially send out its data readings one-by-one. During reads, we have to check timings in order to determine if the sent pulse is denoting a one or zero. If an incoming pulse is high for more than 30µs, it is treated as a one or else it is treated otherwise. Again, it is pretty simple.
value <<= 1; while(DHT11_pin_IN() == LOW); delay_us(30); if(DHT11_pin_IN() == HIGH) { value |= 1; } while(DHT11_pin_IN() == HIGH);
Demo
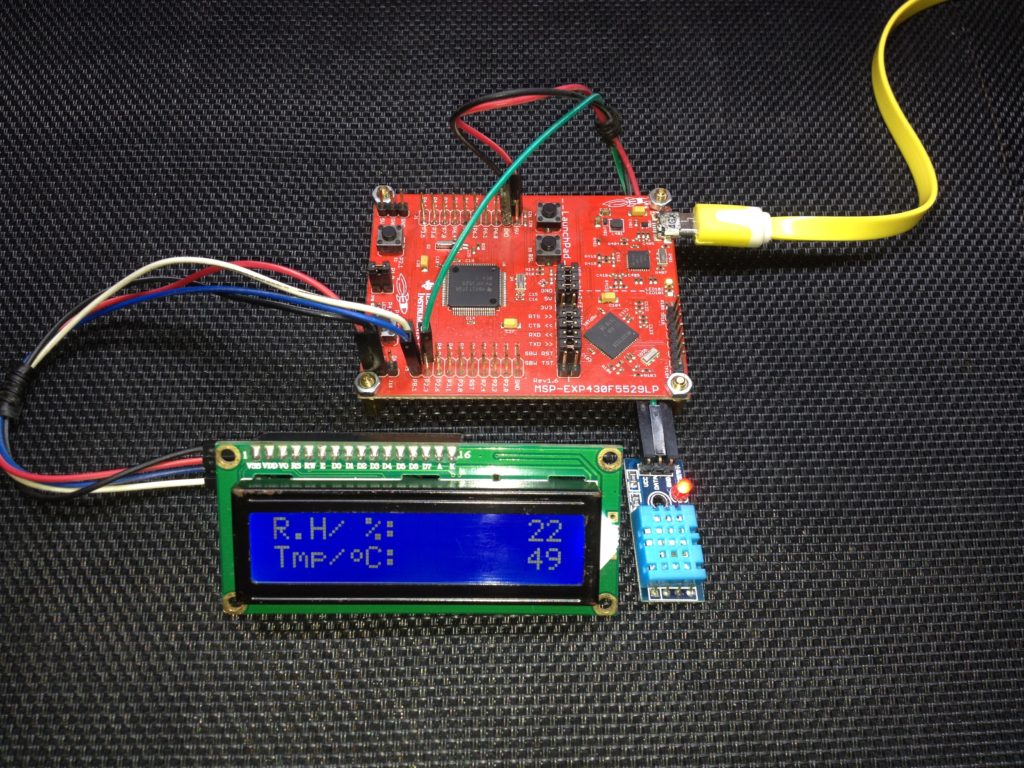
|
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True