Tinkering TI MSP430F5529
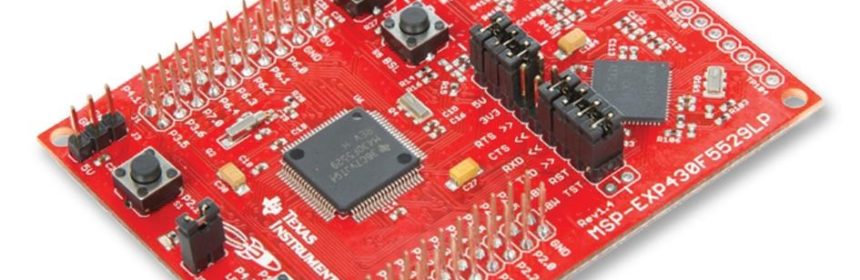
|
RTC_A as Counter
As with other timers, counter mode is a secondary feature of RTC_A module. In this mode, the calendar feature of RTC_A is not used. Instead of being an RTC, RTC_A in this mode behaves like a regular timer. However, unlike other regular timers, we cannot get timer count. This does not limit us much from using it for time bases as there are two counters that can be cascaded and, individually and independently prescaled. RTC_A is rarely used in this role because it is only used as such when we have run out of regular timers and have no need for RTC.
Code Example
#include "driverlib.h" #include "delay.h" void clock_init(void); void GPIO_init(void); void RTC_init(void); #pragma vector=RTC_VECTOR __interrupt void RTC_A_ISR(void) { switch (__even_in_range(RTCIV, 16)) { case 0: break; //No interrupts case 2: break; //RTCRDYIFG case 4: //RTCEVIFG { GPIO_toggleOutputOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_toggleOutputOnPin(GPIO_PORT_P4, GPIO_PIN7); break; } case 6: break; //RTCAIFG case 8: break; //RT0PSIFG case 10: break; //RT1PSIFG default: break; } } void main(void) { WDT_A_hold(WDT_A_BASE); clock_init(); GPIO_init(); RTC_init(); while(1) { }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_3, UCS_XCAP_3); UCS_initClockSignal(UCS_FLLREF, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_4); UCS_initFLLSettle(MCLK_KHZ, MCLK_FLLREF_RATIO); UCS_initClockSignal(UCS_SMCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_ACLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1); } void GPIO_init(void) { GPIO_setAsOutputPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setDriveStrength(GPIO_PORT_P1, GPIO_PIN0, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setAsOutputPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setDriveStrength(GPIO_PORT_P4, GPIO_PIN7, GPIO_FULL_OUTPUT_DRIVE_STRENGTH); GPIO_setOutputLowOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setOutputHighOnPin(GPIO_PORT_P4, GPIO_PIN7); } void RTC_init(void) { RTC_A_initCounter(RTC_A_BASE, RTC_A_CLOCKSELECT_RT1PS, RTC_A_COUNTERSIZE_16BIT); RTC_A_initCounterPrescale(RTC_A_BASE, RTC_A_PRESCALE_0, RTC_A_PSCLOCKSELECT_SMCLK, RTC_A_PSDIVIDER_4); RTC_A_initCounterPrescale(RTC_A_BASE, RTC_A_PRESCALE_1, RTC_A_PSCLOCKSELECT_RT0PS, RTC_A_PSDIVIDER_16); RTC_A_setCounterValue(RTC_A_BASE, 0); RTC_A_clearInterrupt(RTC_A_BASE, RTC_A_TIME_EVENT_INTERRUPT); RTC_A_clearInterrupt(RTC_A_BASE, RTC_A_PRESCALE_TIMER1_INTERRUPT); RTC_A_enableInterrupt(RTC_A_BASE, RTC_A_TIME_EVENT_INTERRUPT); RTC_A_enableInterrupt(RTC_A_BASE, RTC_A_PRESCALE_TIMER1_INTERRUPT); RTC_A_startClock(RTC_A_BASE); __enable_interrupt(); }
Hardware Setup
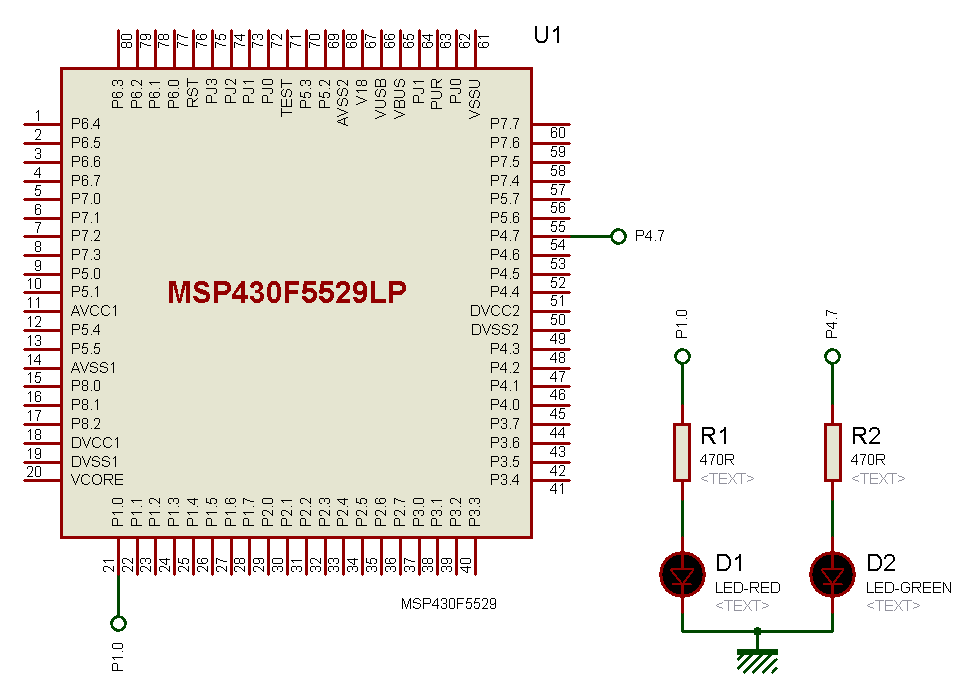
Explanation
For simplest-possible demo, we will again make a LED toggler.
Obviously, counter mode is different from regular calendar mode and so we can use different clock settings. Here, we will be using SMCLK and it will be running at 4 MHz.
UCS_initClockSignal(UCS_SMCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1);
Both on-board LEDs are used but they are initialized at different logic states.
GPIO_setAsOutputPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setAsOutputPin(GPIO_PORT_P4, GPIO_PIN7); GPIO_setOutputLowOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_setOutputHighOnPin(GPIO_PORT_P4, GPIO_PIN7);
RTC_A module’s setup this time is obviously different from the last RTC_A example.
void RTC_init(void) { RTC_A_initCounter(RTC_A_BASE, RTC_A_CLOCKSELECT_RT1PS, RTC_A_COUNTERSIZE_16BIT); RTC_A_initCounterPrescale(RTC_A_BASE, RTC_A_PRESCALE_0, RTC_A_PSCLOCKSELECT_SMCLK, RTC_A_PSDIVIDER_4); RTC_A_initCounterPrescale(RTC_A_BASE, RTC_A_PRESCALE_1, RTC_A_PSCLOCKSELECT_RT0PS, RTC_A_PSDIVIDER_16); RTC_A_setCounterValue(RTC_A_BASE, 0); RTC_A_clearInterrupt(RTC_A_BASE, RTC_A_TIME_EVENT_INTERRUPT); RTC_A_clearInterrupt(RTC_A_BASE, RTC_A_PRESCALE_TIMER1_INTERRUPT); RTC_A_enableInterrupt(RTC_A_BASE, RTC_A_TIME_EVENT_INTERRUPT); RTC_A_enableInterrupt(RTC_A_BASE, RTC_A_PRESCALE_TIMER1_INTERRUPT); RTC_A_startClock(RTC_A_BASE); __enable_interrupt(); }
Firstly, the size of the main RTC counter and its clock source are set. Here the clock source is RT1PS. RT0PS and RT1PS are sub-counters. RT0PS is clocked with either SMCLK or ACLK while RT1PS is clocked with SMCLK, ACLK or prescaled RT0PS. Finally, the RTC module’s main counter can be fed with SMCLK, ACLK or prescaled RT1PS. Thus, here the latter codes involve setting these parameters. RT0PS is fed with 4 MHz SMCLK and then a prescalar of 4 is applied. The resultant frequency is a 1 MHz which is used to feed RT1PS. Now RT1PS is further prescaled by 16, resulting in 62.5 kHz clock. This is what that feeds the main counter. Only 16 bits of the 32-bit main counter is used. Thus, the main counter overflows roughly about every second.
Every overflow event triggers an interrupt. Inside the interrupt on-board LEDs are toggled.
#pragma vector=RTC_VECTOR __interrupt void RTC_A_ISR(void) { switch (__even_in_range(RTCIV, 16)) { case 0: break; //No interrupts case 2: break; //RTCRDYIFG case 4: //RTCEVIFG { GPIO_toggleOutputOnPin(GPIO_PORT_P1, GPIO_PIN0); GPIO_toggleOutputOnPin(GPIO_PORT_P4, GPIO_PIN7); break; } case 6: break; //RTCAIFG case 8: break; //RT0PSIFG case 10: break; //RT1PSIFG default: break; } }
Demo
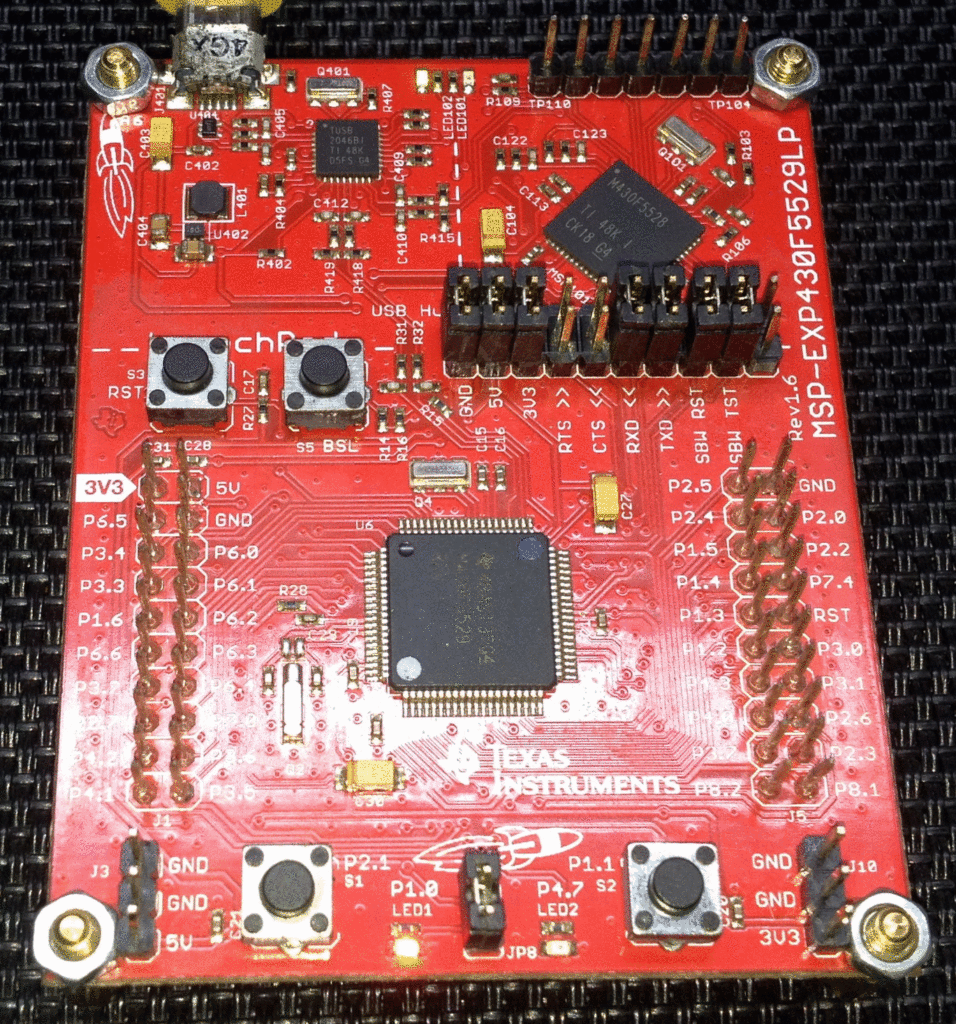
|
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True