Tinkering TI MSP430F5529
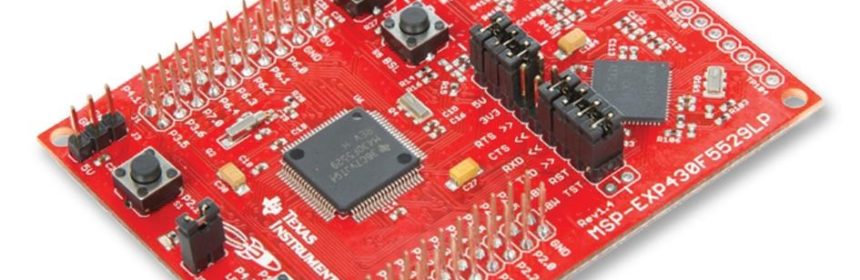
|
Multiple Pulse Width Module (PWM) – TA0
Single PWM is useful for simple purposes but in many applications, we need multiple PWMs. In case of inverters, MOSFET/IGBT drives and bridges, there is no alternative other than to use multi-channel PWM. In this example, we will see how we can use multiple PWM to drive an RGB LED.
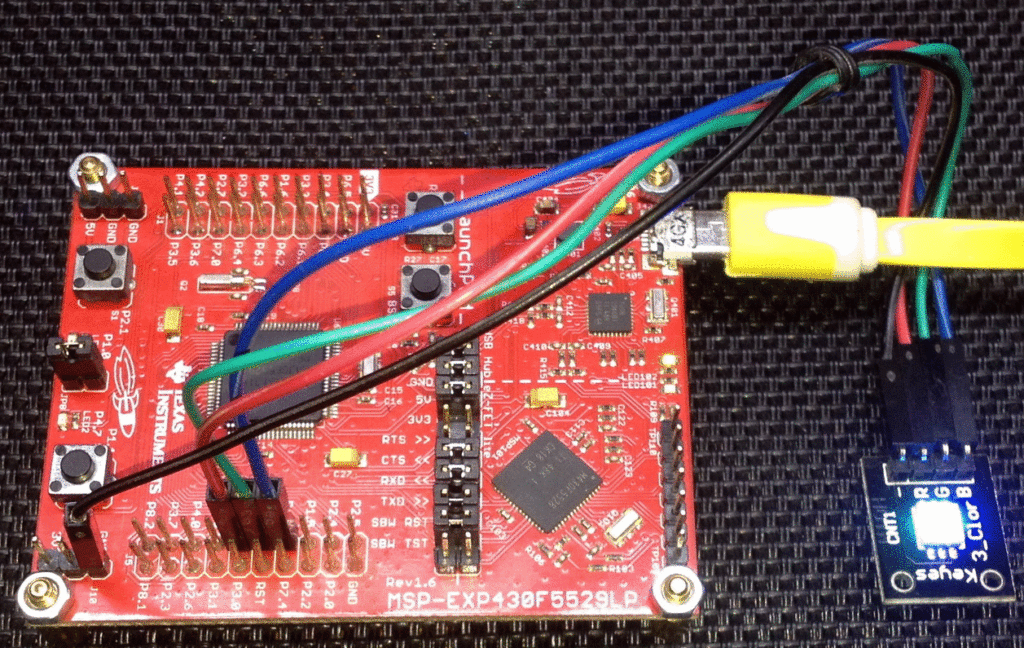
Code Example
#include "driverlib.h" #include "delay.h" #define steps 32 void clock_init(void); void GPIO_init(void); void timer_T0A5_init(void); void main(void) { unsigned char i = 0x00; const unsigned int duty1_value[steps] = {0, 6424, 12786, 19026, 25082, 30896, 36413, 41579, 46344, 50664, 54494, 57801, 60550, 62716, 64278, 65220, 65534, 65218, 64272, 62708, 60540, 57788, 54480, 50647, 46326, 41558, 36391, 30873, 25057, 19000, 12760, 6397}; const unsigned int duty2_value[steps] = {57801, 60550, 62716, 64278, 65220, 65534, 65218, 64272, 62708, 60540, 57788, 54480, 50647, 46326, 41558, 36391, 30873, 25057, 19000, 12760, 6397, 0, 6424, 12786, 19026, 25082, 30896, 36413, 41579, 46344, 50664, 54494}; const unsigned int duty3_value[steps] = {57788, 54480, 50647, 46326, 41558, 36391, 30873, 25057, 19000, 12760, 6397, 0, 6424, 12786, 19026, 25082, 30896, 36413, 41579, 46344, 50664, 54494, 57801, 60550, 62716, 64278, 65220, 65534, 65218, 64272, 62708, 60540}; WDT_A_hold(WDT_A_BASE); clock_init(); GPIO_init(); timer_T0A5_init(); while(true) { for(i = 0; i < steps; i++) { Timer_A_setCompareValue(TIMER_A0_BASE, TIMER_A_CAPTURECOMPARE_REGISTER_1, duty1_value[i]); Timer_A_setCompareValue(TIMER_A0_BASE, TIMER_A_CAPTURECOMPARE_REGISTER_2, duty2_value[i]); Timer_A_setCompareValue(TIMER_A0_BASE, TIMER_A_CAPTURECOMPARE_REGISTER_3, duty3_value[i]); delay_ms(250); } }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_0, UCS_XCAP_3); UCS_initClockSignal(UCS_MCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_SMCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_ACLK, UCS_XT1CLK_SELECT, UCS_CLOCK_DIVIDER_1); } void GPIO_init(void) { GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P1, (GPIO_PIN2 + GPIO_PIN3 + GPIO_PIN4)); } void timer_T0A5_init(void) { Timer_A_initCompareModeParam CompareModeParam1 = {0}; Timer_A_initCompareModeParam CompareModeParam2 = {0}; Timer_A_initCompareModeParam CompareModeParam3 = {0}; Timer_A_initContinuousModeParam ContinuousModeParam = {0}; ContinuousModeParam.clockSource = TIMER_A_CLOCKSOURCE_SMCLK; ContinuousModeParam.clockSourceDivider = TIMER_A_CLOCKSOURCE_DIVIDER_1; ContinuousModeParam.timerClear = TIMER_A_DO_CLEAR; ContinuousModeParam.timerInterruptEnable_TAIE = TIMER_A_TAIE_INTERRUPT_DISABLE; ContinuousModeParam.startTimer = false; CompareModeParam1.compareInterruptEnable = TIMER_A_CCIE_CCR0_INTERRUPT_DISABLE; CompareModeParam1.compareOutputMode = TIMER_A_OUTPUTMODE_RESET_SET; CompareModeParam1.compareRegister = TIMER_A_CAPTURECOMPARE_REGISTER_1; CompareModeParam1.compareValue = 0; CompareModeParam2.compareInterruptEnable = TIMER_A_CCIE_CCR0_INTERRUPT_DISABLE; CompareModeParam2.compareOutputMode = TIMER_A_OUTPUTMODE_RESET_SET; CompareModeParam2.compareRegister = TIMER_A_CAPTURECOMPARE_REGISTER_2; CompareModeParam2.compareValue = 0; CompareModeParam3.compareInterruptEnable = TIMER_A_CCIE_CCR0_INTERRUPT_DISABLE; CompareModeParam3.compareOutputMode = TIMER_A_OUTPUTMODE_RESET_SET; CompareModeParam3.compareRegister = TIMER_A_CAPTURECOMPARE_REGISTER_3; CompareModeParam3.compareValue = 0; Timer_A_initCompareMode(TIMER_A0_BASE, &CompareModeParam1); Timer_A_initCompareMode(TIMER_A0_BASE, &CompareModeParam2); Timer_A_initCompareMode(TIMER_A0_BASE, &CompareModeParam3); Timer_A_initContinuousMode(TIMER_A0_BASE, &ContinuousModeParam); Timer_A_startCounter(TIMER_A0_BASE, TIMER_A_CONTINUOUS_MODE); }
Hardware Setup
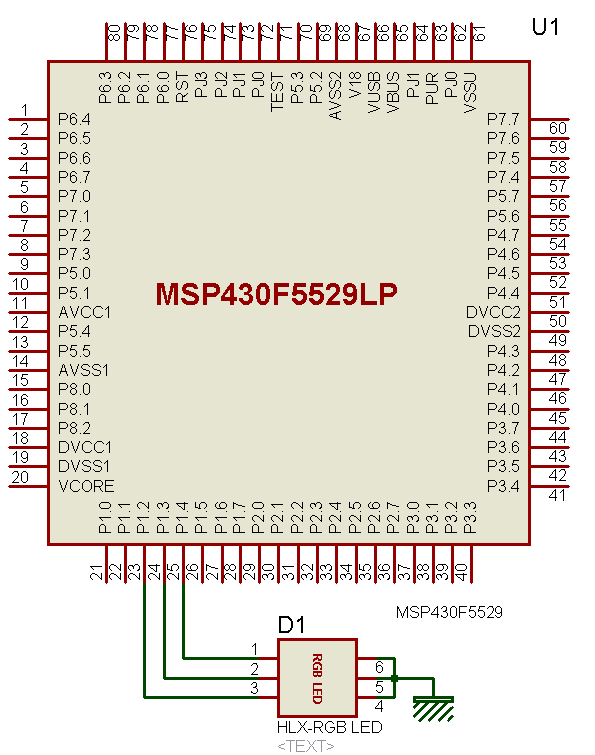
Explanation
Multi-PWM example here uses the same concepts as in the single PWM example. This time timer TA0 is used and the following pins are set for PWM output:
GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P1, (GPIO_PIN2 + GPIO_PIN3 + GPIO_PIN4));
Note that this timer has 5 CC channels associated with it but we are using only three of them since we have connected an RGB LED to these pins.
4MHz XT2CLK source feeds SMCLK which in turn is used to run timer TA0. Thus, timer TA0 is operating at 4MHz speed.
UCS_initClockSignal(UCS_SMCLK, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_1);
Timer PWM setup is not very much different. The only differences are timer mode, the number of channels and their individual settings.
void timer_T0A5_init(void) { Timer_A_initCompareModeParam CompareModeParam1 = {0}; Timer_A_initCompareModeParam CompareModeParam2 = {0}; Timer_A_initCompareModeParam CompareModeParam3 = {0}; Timer_A_initContinuousModeParam ContinuousModeParam = {0}; ContinuousModeParam.clockSource = TIMER_A_CLOCKSOURCE_SMCLK; ContinuousModeParam.clockSourceDivider = TIMER_A_CLOCKSOURCE_DIVIDER_1; ContinuousModeParam.timerClear = TIMER_A_DO_CLEAR; ContinuousModeParam.timerInterruptEnable_TAIE = TIMER_A_TAIE_INTERRUPT_DISABLE; ContinuousModeParam.startTimer = false; CompareModeParam1.compareInterruptEnable = TIMER_A_CCIE_CCR0_INTERRUPT_DISABLE; CompareModeParam1.compareOutputMode = TIMER_A_OUTPUTMODE_RESET_SET; CompareModeParam1.compareRegister = TIMER_A_CAPTURECOMPARE_REGISTER_1; CompareModeParam1.compareValue = 0; CompareModeParam2.compareInterruptEnable = TIMER_A_CCIE_CCR0_INTERRUPT_DISABLE; CompareModeParam2.compareOutputMode = TIMER_A_OUTPUTMODE_RESET_SET; CompareModeParam2.compareRegister = TIMER_A_CAPTURECOMPARE_REGISTER_2; CompareModeParam2.compareValue = 0; CompareModeParam3.compareInterruptEnable = TIMER_A_CCIE_CCR0_INTERRUPT_DISABLE; CompareModeParam3.compareOutputMode = TIMER_A_OUTPUTMODE_RESET_SET; CompareModeParam3.compareRegister = TIMER_A_CAPTURECOMPARE_REGISTER_3; CompareModeParam3.compareValue = 0; Timer_A_initCompareMode(TIMER_A0_BASE, &CompareModeParam1); Timer_A_initCompareMode(TIMER_A0_BASE, &CompareModeParam2); Timer_A_initCompareMode(TIMER_A0_BASE, &CompareModeParam3); Timer_A_initContinuousMode(TIMER_A0_BASE, &ContinuousModeParam); Timer_A_startCounter(TIMER_A0_BASE, TIMER_A_CONTINUOUS_MODE); }
Unlike in the previous PWM example, timer TA0 is set for continuous mode of operation and so the top PWM count value is 65535. Thus, the period of the PWMs is about 16 ms. No interrupt is used. We just have to setup each channel individually and then run the timer in continuous mode.
In the main loop, PWMs of each channel are altered and the effect is visible in the form of RGB LED’s changing colour.
for(i = 0; i < steps; i++) { Timer_A_setCompareValue(TIMER_A0_BASE, TIMER_A_CAPTURECOMPARE_REGISTER_1, duty1_value[i]); Timer_A_setCompareValue(TIMER_A0_BASE, TIMER_A_CAPTURECOMPARE_REGISTER_2, duty2_value[i]); Timer_A_setCompareValue(TIMER_A0_BASE, TIMER_A_CAPTURECOMPARE_REGISTER_3, duty3_value[i]); delay_ms(250); }
The duty cycle of each PWM channel is coded to in a half-sine wave pattern but each of them has a different phase shift from the other.
const unsigned int duty3_value[steps] = {57788, 54480, 50647, 46326, 41558, 36391, 30873, 25057, 19000, 12760, 6397, 0, 6424, 12786, 19026, 25082, 30896, 36413, 41579, 46344, 50664, 54494, 57801, 60550, 62716, 64278, 65220, 65534, 65218, 64272, 62708, 60540};
When combined with the three LEDs of the RGB, this creates a beautiful rhythmic color pattern.
Demo
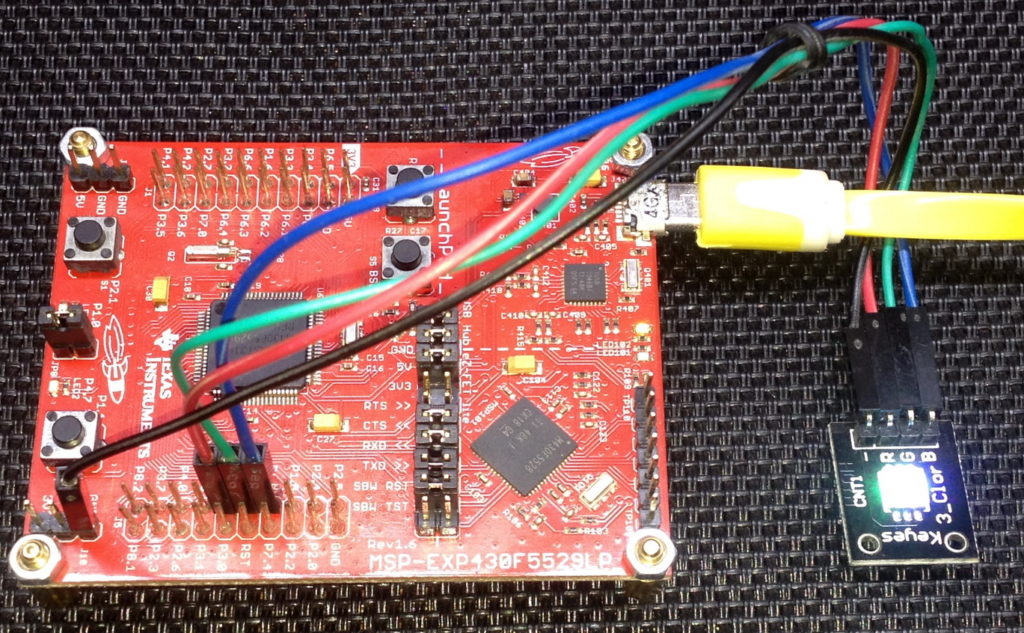
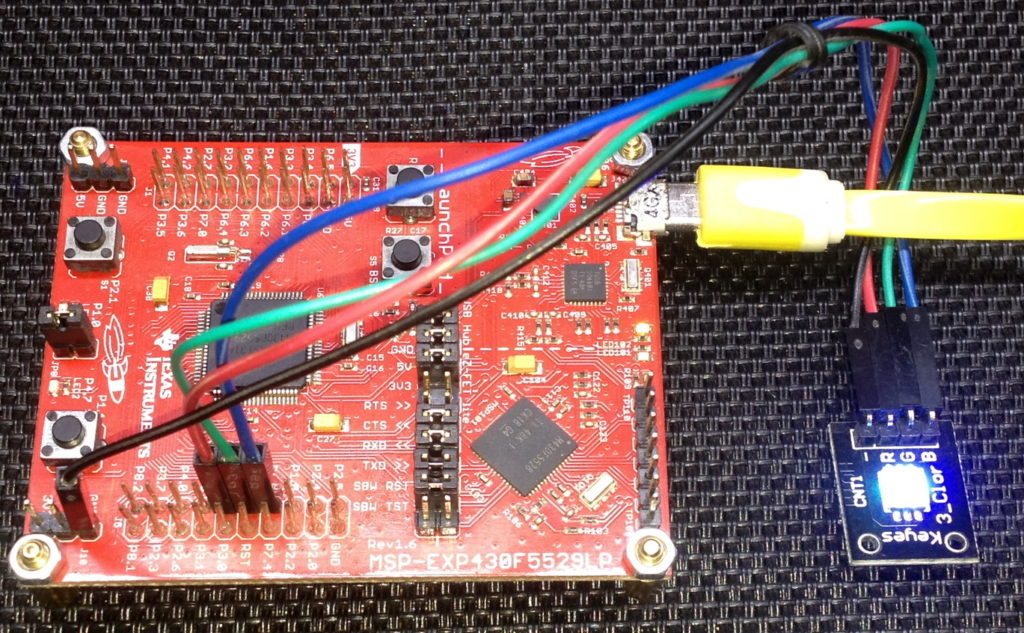
|
Hello, what software are you using for the Hardware setup images and does it support simulation for the MSP430F5529
I used Proteus VSM for drawing schematics but it doesn’t support simulation.
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True