Tinkering TI MSP430F5529
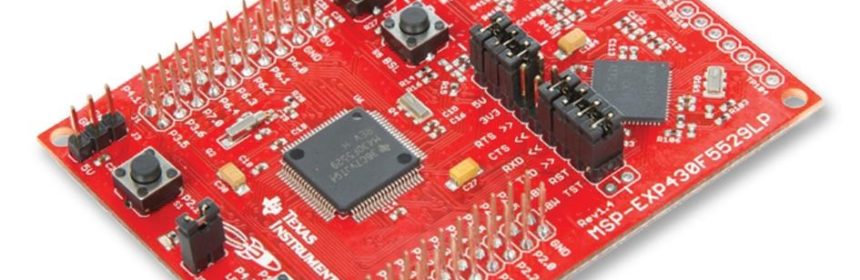
|
USB HID
Most of the common devices like keyboards, mice, printers, scanners, barcode readers, gamepads, etc that we use with our PCs and laptops in regular life are USB HID devices. We can use our MSP430F5529 microcontroller to make USB HID devices and in this example, we will use our MSP430 microcontroller to make a rudimentary mouse. Unlike conventional mice, our mouse will be using a keypad joystick instead of optoelectronics or mechanical trackball.
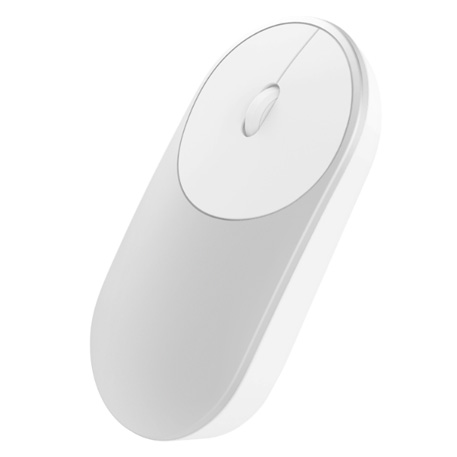
Code Example
#include "driverlib.h" #include "delay.h" #include "lcd.h" #include "lcd_print.h" #include "USB_config/descriptors.h" #include "USB_API/USB_Common/device.h" #include "USB_API/USB_Common/usb.h" #include "USB_API/USB_HID_API/UsbHid.h" #include "hal.h" #define LED_PORT GPIO_PORT_P4 #define LED_PIN GPIO_PIN7 #define MOUSE_PORT GPIO_PORT_P6 #define MOUSE_UP_PIN GPIO_PIN0 #define MOUSE_DOWN_PIN GPIO_PIN1 #define MOUSE_LEFT_PIN GPIO_PIN2 #define MOUSE_RIGHT_PIN GPIO_PIN3 #define MOUSE_BUTTON_PIN GPIO_PIN4 #define change_amount 1 typedef struct { int8_t buttons; int8_t dX; int8_t dY; int8_t dZ; }MOUSE_REPORT; MOUSE_REPORT mouseReport = {0x00, 0x00, 0x00, 0x00}; void DIO_init(void); #pragma vector = UNMI_VECTOR __interrupt void UNMI_ISR (void) { switch (__even_in_range(SYSUNIV, SYSUNIV_BUSIFG )) { case SYSUNIV_NONE: { __no_operation(); break; } case SYSUNIV_NMIIFG: { __no_operation(); break; } case SYSUNIV_OFIFG: { UCS_clearFaultFlag(UCS_XT2OFFG); UCS_clearFaultFlag(UCS_DCOFFG); SFR_clearInterrupt(SFR_OSCILLATOR_FAULT_INTERRUPT); break; } case SYSUNIV_ACCVIFG: { __no_operation(); break; } case SYSUNIV_BUSIFG: { SYSBERRIV = 0; USB_disable(); } } } void main (void) { unsigned char t = 0x0000; WDT_A_hold(WDT_A_BASE); LCD_init(); LCD_clear_home(); LCD_goto(1, 0); LCD_putstr("MSP430 USB HID"); LCD_goto(0, 1); LCD_putstr("X: "); LCD_goto(6, 1); LCD_putstr("Y:"); LCD_goto(12, 1); LCD_putstr("B:"); PMM_setVCore(PMM_CORE_LEVEL_2); USBHAL_initPorts(); USBHAL_initClocks(8000000); USB_setup(TRUE, TRUE); DIO_init(); __enable_interrupt(); while (1) { if(GPIO_getInputPinValue(MOUSE_PORT, MOUSE_UP_PIN) == false) { mouseReport.dY += change_amount; } if(GPIO_getInputPinValue(MOUSE_PORT, MOUSE_DOWN_PIN) == false) { mouseReport.dY -= change_amount; } if(mouseReport.dY > 127) { mouseReport.dY = 127; } if(mouseReport.dY 127) { mouseReport.dX = 127; } if(mouseReport.dX 40) { t = 0; mouseReport.dX = 0; mouseReport.dY = 0; mouseReport.buttons = 0; } } } void DIO_init(void) { GPIO_setAsInputPinWithPullUpResistor(MOUSE_PORT, MOUSE_UP_PIN); GPIO_setAsInputPinWithPullUpResistor(MOUSE_PORT, MOUSE_DOWN_PIN); GPIO_setAsInputPinWithPullUpResistor(MOUSE_PORT, MOUSE_LEFT_PIN); GPIO_setAsInputPinWithPullUpResistor(MOUSE_PORT, MOUSE_RIGHT_PIN); GPIO_setAsInputPinWithPullUpResistor(MOUSE_PORT, MOUSE_BUTTON_PIN); }
Hardware Setup
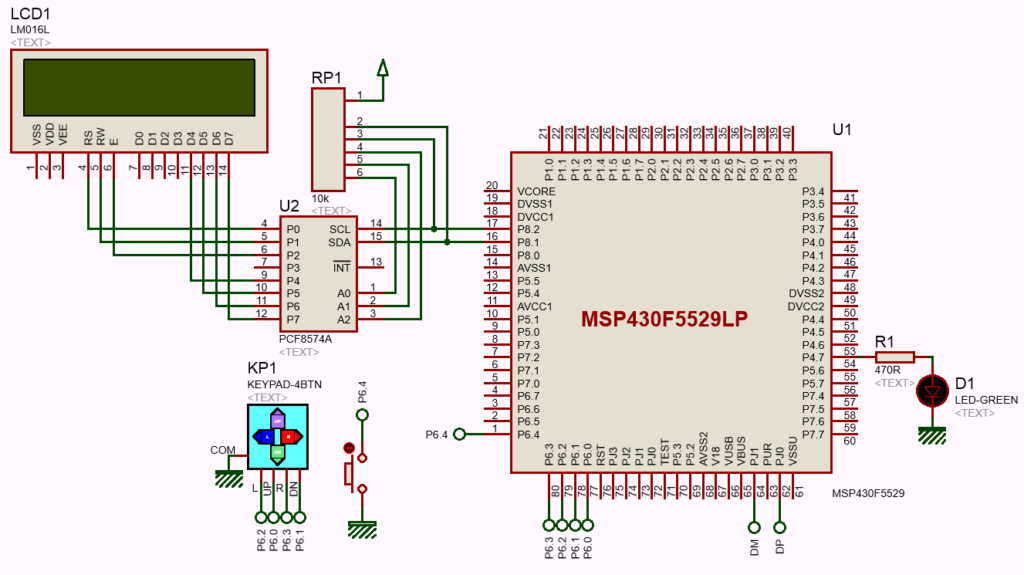
Explanation
Just like the past example, again we are interested in the main loop as everything is done here. On each press of joystick buttons x and y coordinates are changed by 1 unit. We are not using mouse coordinate acceleration as with any regular mouse and that is why the change amount is 1 unit only. On PC screen, the mouse cursor will appear to move slowly as if the PC is busy doing some other works. We are also assuming that the x and y coordinate boundaries range from -127 to +127 units. In the code, we have to take care that these extremes are not exceeded. We are not using typical mouse click buttons although I coded the joystick middle button for clicking. This is not very important here as mouse cursor movement is all that is needed for USB HID demo. In the ST_ENUM_ACTIVE case, the location of cursor and button state are reported to host PC. The PC then uses these to change the position of the mouse cursor.
while (1) { if(GPIO_getInputPinValue(MOUSE_PORT, MOUSE_UP_PIN) == false) { mouseReport.dY += change_amount; } if(GPIO_getInputPinValue(MOUSE_PORT, MOUSE_DOWN_PIN) == false) { mouseReport.dY -= change_amount; } if(mouseReport.dY > 127) { mouseReport.dY = 127; } if(mouseReport.dY 127) { mouseReport.dX = 127; } if(mouseReport.dX 40) { t = 0; mouseReport.dX = 0; mouseReport.dY = 0; mouseReport.buttons = 0; } }
For 40 times, the mouse’s coordinate changes and button states are not reset automatically and this is so because we don’t want to keep holding the joystick buttons for movement once our desired speed is achieved. Remember no acceleration is used.
Demo
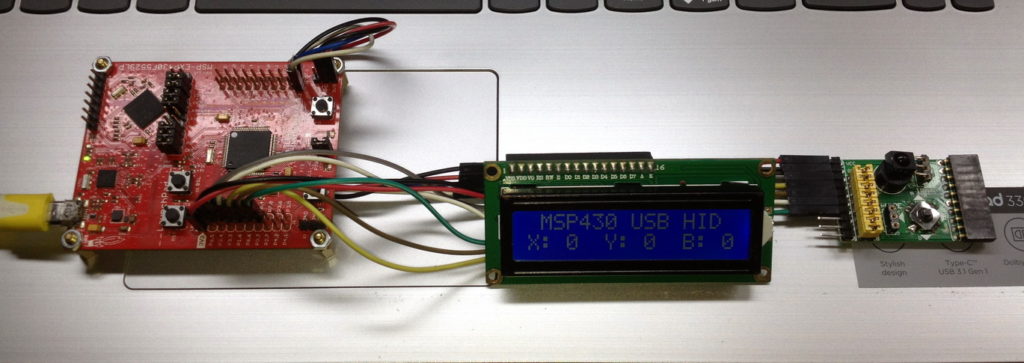
|
Hello, what software are you using for the Hardware setup images and does it support simulation for the MSP430F5529
I used Proteus VSM for drawing schematics but it doesn’t support simulation.
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True