Tinkering TI MSP430F5529
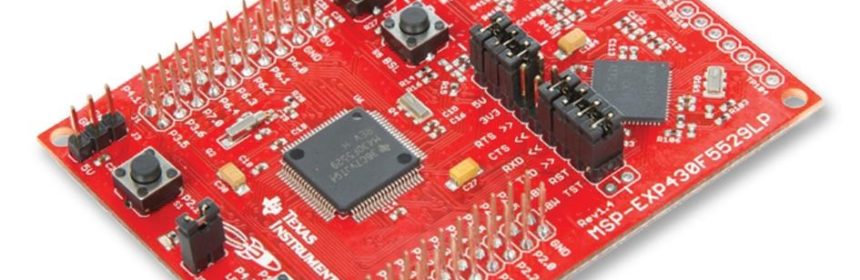
|
2 Wire (Software I2C) LCD
Alphanumerical LCDs need lot of pins (6 pins at least) for interfacing and in some cases, this is an expensive requirement. In such cases, hardware designs become complex and every pin is precious for their secondary roles. We can avoid this by using software-based I2C or SPI LCD drivers.
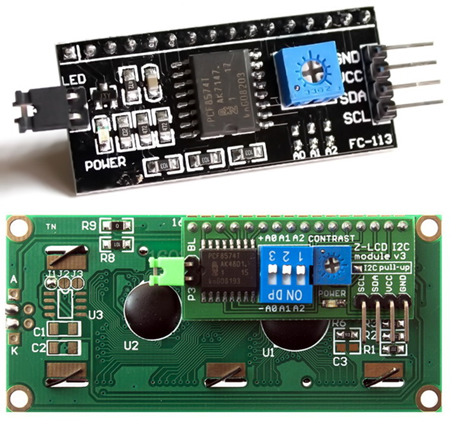
We can use I2C-based port expanders like PCF8574 or MCP23017 for this purpose.
Code Example
SW_I2C.h
#include "driverlib.h" #include "delay.h" #define SW_I2C_SDA_PORT GPIO_PORT_P8 #define SW_I2C_SCL_PORT GPIO_PORT_P8 #define SDA_pin GPIO_PIN1 #define SCL_pin GPIO_PIN2 #define SDA_DIR_OUT() GPIO_setAsOutputPin(SW_I2C_SDA_PORT, SDA_pin) #define SDA_DIR_IN() GPIO_setAsInputPin(SW_I2C_SDA_PORT, SDA_pin) #define SCL_DIR_OUT() GPIO_setAsOutputPin(SW_I2C_SCL_PORT, SCL_pin) #define SCL_DIR_IN() GPIO_setAsInputPin(SW_I2C_SCL_PORT, SCL_pin) #define SDA_HIGH() GPIO_setOutputHighOnPin(SW_I2C_SDA_PORT, SDA_pin) #define SDA_LOW() GPIO_setOutputLowOnPin(SW_I2C_SDA_PORT, SDA_pin) #define SCL_HIGH() GPIO_setOutputHighOnPin(SW_I2C_SCL_PORT, SCL_pin) #define SCL_LOW() GPIO_setOutputLowOnPin(SW_I2C_SCL_PORT, SCL_pin) #define SDA_IN() GPIO_getInputPinValue(SW_I2C_SDA_PORT, SDA_pin) #define I2C_ACK 0xFF #define I2C_NACK 0x00 #define I2C_timeout 1000 void SW_I2C_init(void); void SW_I2C_start(void); void SW_I2C_stop(void); unsigned char SW_I2C_read(unsigned char ack); void SW_I2C_write(unsigned char value); void SW_I2C_ACK_NACK(unsigned char mode); unsigned char SW_I2C_wait_ACK(void);
SW_I2C.c
#include "SW_I2C.h" void SW_I2C_init(void) { SDA_DIR_OUT(); SCL_DIR_OUT(); delay_ms(1); SDA_HIGH(); SCL_HIGH(); } void SW_I2C_start(void) { SDA_DIR_OUT(); SDA_HIGH(); SCL_HIGH(); delay_us(4); SDA_LOW(); delay_us(4); SCL_LOW(); } void SW_I2C_stop(void) { SDA_DIR_OUT(); SDA_LOW(); SCL_LOW(); delay_us(4); SDA_HIGH(); SCL_HIGH(); delay_us(4); } unsigned char SW_I2C_read(unsigned char ack) { unsigned char i = 8; unsigned char j = 0; SDA_DIR_IN(); while(i > 0) { SCL_LOW(); delay_us(2); SCL_HIGH(); delay_us(2); j < 0) { if(((value & 0x80) >> 7) != 0x00) { SDA_HIGH(); } else { SDA_LOW(); } value < I2C_timeout) { SW_I2C_stop(); return 1; } }; SCL_LOW(); return 0; }
PCF8574.h
#include "SW_I2C.h" #define PCF8574_address 0x4E #define PCF8574_write_cmd PCF8574_address #define PCF8574_read_cmd (PCF8574_address + 1) void PCF8574_init(void); unsigned char PCF8574_read(void); void PCF8574_write(unsigned char data_byte);
PCF8574.c
#include "PCF8574.h" void PCF8574_init(void) { SW_I2C_init(); delay_ms(100); } unsigned char PCF8574_read(void) { unsigned char port_byte = 0; SW_I2C_start(); SW_I2C_write(PCF8574_read_cmd); port_byte = SW_I2C_read(I2C_NACK); SW_I2C_stop(); return port_byte; } void PCF8574_write(unsigned char data_byte) { SW_I2C_start(); SW_I2C_write(PCF8574_write_cmd); SW_I2C_ACK_NACK(I2C_ACK); SW_I2C_write(data_byte); SW_I2C_ACK_NACK(I2C_ACK); SW_I2C_stop(); }
lcd.h
#include "PCF8574.h" #define clear_display 0x01 #define goto_home 0x02 #define cursor_direction_inc (0x04 | 0x02) #define cursor_direction_dec (0x04 | 0x00) #define display_shift (0x04 | 0x01) #define display_no_shift (0x04 | 0x00) #define display_on (0x08 | 0x04) #define display_off (0x08 | 0x02) #define cursor_on (0x08 | 0x02) #define cursor_off (0x08 | 0x00) #define blink_on (0x08 | 0x01) #define blink_off (0x08 | 0x00) #define _8_pin_interface (0x20 | 0x10) #define _4_pin_interface (0x20 | 0x00) #define _2_row_display (0x20 | 0x08) #define _1_row_display (0x20 | 0x00) #define _5x10_dots (0x20 | 0x40) #define _5x7_dots (0x20 | 0x00) #define BL_ON 1 #define BL_OFF 0 #define dly 2 #define DAT 1 #define CMD 0 void LCD_init(void); void LCD_toggle_EN(void); void LCD_send(unsigned char value, unsigned char mode); void LCD_4bit_send(unsigned char lcd_data); void LCD_putstr(char *lcd_string); void LCD_putchar(char char_data); void LCD_clear_home(void); void LCD_goto(unsigned char x_pos, unsigned char y_pos);
lcd.c
#include "lcd.h" static unsigned char bl_state; static unsigned char data_value; void LCD_init(void) { PCF8574_init(); delay_ms(100); bl_state = BL_ON; data_value = 0x04; PCF8574_write(data_value); delay_ms(10); LCD_send(0x33, CMD); LCD_send(0x32, CMD); LCD_send((_4_pin_interface | _2_row_display | _5x7_dots), CMD); LCD_send((display_on | cursor_off | blink_off), CMD); LCD_send((clear_display), CMD); LCD_send((cursor_direction_inc | display_no_shift), CMD); } void LCD_toggle_EN(void) { data_value |= 0x04; PCF8574_write(data_value); delay_ms(dly); data_value &= 0xF9; PCF8574_write(data_value); delay_ms(dly); } void LCD_send(unsigned char value, unsigned char mode) { switch(mode) { case CMD: { data_value &= 0xF4; break; } case DAT: { data_value |= 0x01; break; } } switch(bl_state) { case BL_ON: { data_value |= 0x08; break; } case BL_OFF: { data_value &= 0xF7; break; } } PCF8574_write(data_value); LCD_4bit_send(value); delay_ms(dly); } void LCD_4bit_send(unsigned char lcd_data) { unsigned char temp = 0x00; temp = (lcd_data & 0xF0); data_value &= 0x0F; data_value |= temp; PCF8574_write(data_value); LCD_toggle_EN(); temp = (lcd_data & 0x0F); temp <= 0x20) && (char_data <= 0x7F)) { LCD_send(char_data, DAT); } } void LCD_clear_home(void) { LCD_send(clear_display, CMD); LCD_send(goto_home, CMD); } void LCD_goto(unsigned char x_pos,unsigned char y_pos) { if(y_pos == 0) { LCD_send((0x80 | x_pos), CMD); } else { LCD_send((0x80 | 0x40 | x_pos), CMD); } }
main.c
#include "driverlib.h" #include "delay.h" #include "lcd.h" void clock_init(void); void show_value(unsigned char value); void main(void) { unsigned char s = 0x00; char txt1[] = {"MICROARENA"}; char txt2[] = {"SShahryiar"}; char txt3[] = {"MSP430F5529LP"}; char txt4[] = {"Launchpad!"}; WDT_A_hold(WDT_A_BASE); clock_init(); LCD_init(); LCD_clear_home(); LCD_goto(3, 0); LCD_putstr(txt1); LCD_goto(3, 1); LCD_putstr(txt2); delay_ms(4000); LCD_clear_home(); for(s = 0; s < 13; s++) { LCD_goto((1 + s), 0); LCD_putchar(txt3[s]); delay_ms(60); } for(s = 0; s < 10; s++) { LCD_goto((3 + s), 1); LCD_putchar(txt4[s]); delay_ms(60); } delay_ms(4000); s = 0; LCD_clear_home(); LCD_goto(3, 0); LCD_putstr(txt1); while(1) { show_value(s); s++; delay_ms(400); }; } void clock_init(void) { PMM_setVCore(PMM_CORE_LEVEL_3); GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P5, (GPIO_PIN4 | GPIO_PIN2)); GPIO_setAsPeripheralModuleFunctionOutputPin(GPIO_PORT_P5, (GPIO_PIN5 | GPIO_PIN3)); UCS_setExternalClockSource(XT1_FREQ, XT2_FREQ); UCS_turnOnXT2(UCS_XT2_DRIVE_4MHZ_8MHZ); UCS_turnOnLFXT1(UCS_XT1_DRIVE_0, UCS_XCAP_3); UCS_initClockSignal(UCS_FLLREF, UCS_XT2CLK_SELECT, UCS_CLOCK_DIVIDER_4); UCS_initFLLSettle(MCLK_KHZ, MCLK_FLLREF_RATIO); UCS_initClockSignal(UCS_SMCLK, UCS_REFOCLK_SELECT, UCS_CLOCK_DIVIDER_1); UCS_initClockSignal(UCS_ACLK, UCS_XT1CLK_SELECT, UCS_CLOCK_DIVIDER_1); } void show_value(unsigned char value) { unsigned char ch = 0x00; ch = ((value / 100) + 0x30); LCD_goto(6, 1); LCD_putchar(ch); ch = (((value / 10) % 10) + 0x30); LCD_goto(7, 1); LCD_putchar(ch); ch = ((value % 10) + 0x30); LCD_goto(8, 1); LCD_putchar(ch); }
Hardware Setup
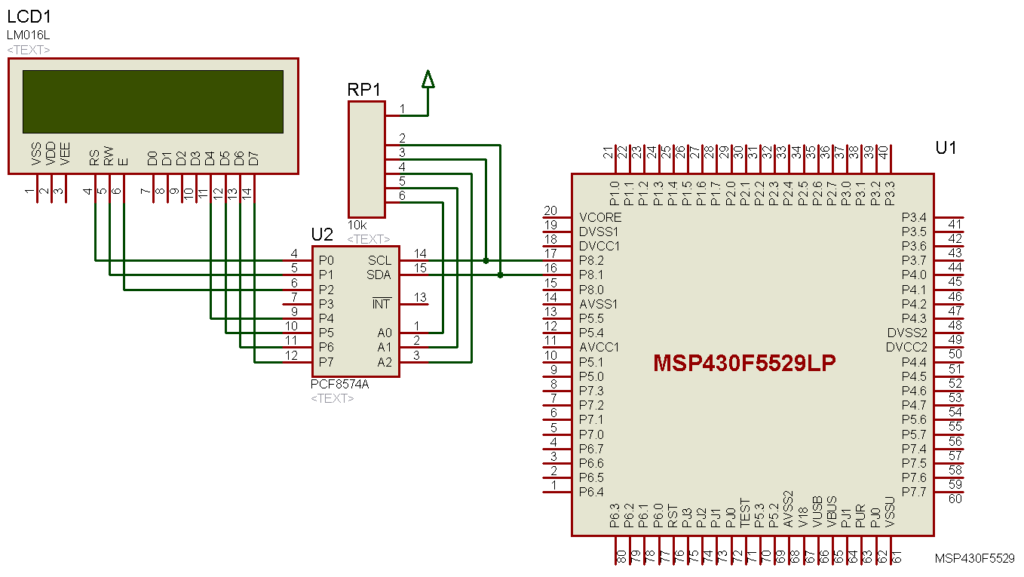
Explanation
The initialization and working procedure of the LCD module is nothing different from the first LCD example. The only exception is the fact that a PCF8574 port expander is used to manipulate the LCD I/Os instead of direct host micro connection.
PCF8574 from NXP is an I2C-based 8-bit port expander. With just two GPIO pins or I2C pins we can read and write a complete external 8-bit GPIO port. This is what makes PCF8574 a GPIO expander.
Since we are yet to check out the hardware I2C option of MSP430F5529 micro, we will be using software-based I2C. The software I2C driver is coded in SW_I2Cheader and source files. The lines of code there are self-explanatory. All of the I2C operations are simulated by altering two GPIO pin states and using software delays. The important part, however, is the GPIO declarations and it is found in the following part of the SW_I2C header file.
#define SW_I2C_SDA_PORT GPIO_PORT_P8 #define SW_I2C_SCL_PORT GPIO_PORT_P8 #define SDA_pin GPIO_PIN1 #define SCL_pin GPIO_PIN2
We are not going to read our LCD module and so we don’t need PCF8574_read function of PCF8574 library. We’ll just need the write function.
void PCF8574_write(unsigned char data_byte) { SW_I2C_start(); SW_I2C_write(PCF8574_write_cmd); SW_I2C_ACK_NACK(I2C_ACK); SW_I2C_write(data_byte); SW_I2C_ACK_NACK(I2C_ACK); SW_I2C_stop(); }
We send the address and command of the PCF8574 module first and then we send the port states. Note that the port states are sent just like the first LCD example.
Demo
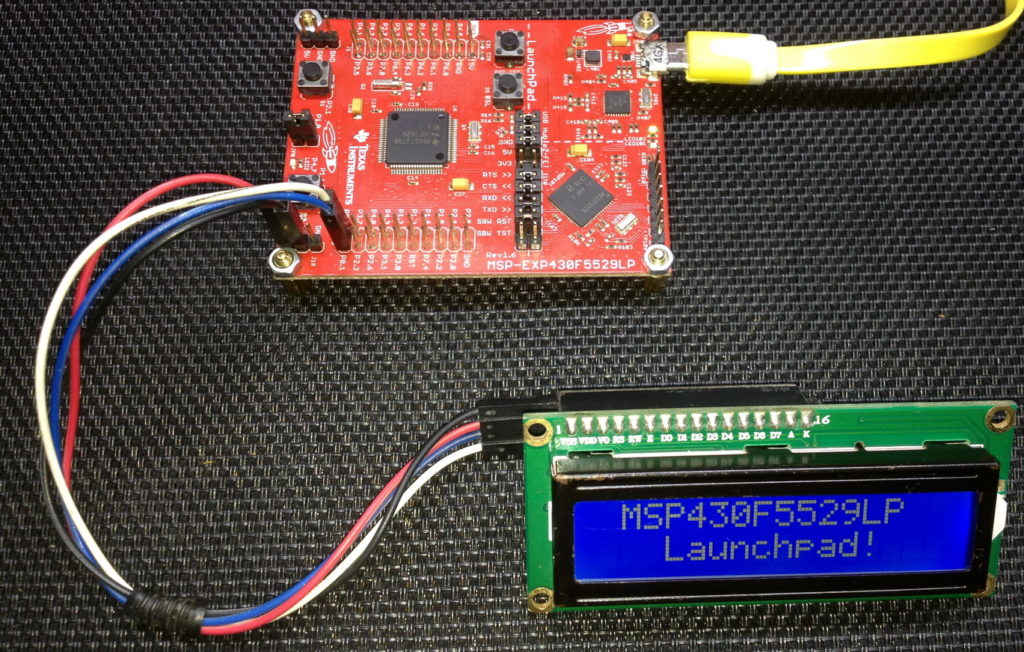
|
Hello, what software are you using for the Hardware setup images and does it support simulation for the MSP430F5529
I used Proteus VSM for drawing schematics but it doesn’t support simulation.
Hi,
Im interfacing MSP430F5529 with MAX17055 fuel guage. while reading 16 bit value, the first byte im receiving is 0. so while reading multiple registers continuously the data exchange is happening, but im getting the correct data. Can anyone suggest me what will be the issue? why im getting 0 in first byte?
read16_bit data code:
uint16_t value = 0;
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterSendStart(USCI_B1_BASE);
while (!USCI_B_I2C_masterSendStart(USCI_B1_BASE));
USCI_B_I2C_mastterSendSingleByte(USCI_B1_BASE, reg_address);
USCI_B_I2C_setslaveaddress(USCI_B1_BASE, slave_address);
USCI_B_I2C_setmode(USCI_B1_BASE, USCI_B_I2C_TRANSMIT_MODE);
USCI_B_I2C_masterReceiveMultiByteStart(USCI_B1_BASE);
uint8_t lb = USCI_B_I2C_masterReceiveMultiByteNext(USCI_B1_BASE);
uint8_t hb = USCI_B_I2C_masterReceiveMultiByteFinish(USCI_B1_BASE);
while (USCI_B_I2C_isBusBusy(USCI_B_BASE));
value = lb << 8;
value |= hb;
return value;
In code, after sending reg address, it will be recieve mode. its a type mistake
Hi, im trying to send the command from the terminal view. i can able to send the command and tried to blink p1.0 led in msp430f5529 controller, its working fine. And im using led driver IS31FL3236A interfaced with msp430f5529 controller, i can able to interface im getting the expected output.
now i need to send the command from seriak monitor based on that command i2c communication need to start. both communication are working fine, when it runs separately. its not working when i tried to combine.
any one had any idea, why it is happening or what will be the issue?
It could be due to:
1. conflicts in clock settings
2. hardware conflict like pin mapping
3. code is getting stuck or waiting for one communication line to finish
4. use of polling method instead of interrupt-driven coding
Hi, thank you for the respose.
Do I need to use different clock initialization for I2C and UART communication? if YES, can you explain how to do that?
I mean check which clock has been set for UART and I2C…. Is it SMCLK, MCLK, etc and is it tuned to right frequency required by the respective hardware?
Is there any example on how to implement polling method in uart?
Why go for polling method when it is a blocking method of coding? It is better to use interrupts instead at least for UART receive.
yes!! currently in my code, only for uart im using interrupts to recieve command from serial monitor. Im not using interrupt for I2C communication.
so the issue is must be in clock initialization. right?
For UART, im using USCI_A1_BASE. and for I2C, im using USCI_B1_BASE.
And another thing i need to ask is, in uart when i tried blink led(p1.0) in msp430f5529 by passing command. here, without clock I’m getting output. how it is possible?
And for both i2c and uart i gave SMCLK with 1Mhz
I am surprised and happy to find this tutorial on the F5529 as TI makes a lot of different devices.
Thank you very much for putting in the extra knowledge in each segment, made reading worthwhile.
Good Work!
lovely tutorial but to be honest I don’t think I’d be investing my time on this board to start with it’s not cheap and readily available as the stm32 boards can you please do more tutorials on stm32 board’s and the stc micros thanks
Hello, I try to program MSP430FR6047 but i get error “the debug interface to the device has been secured”. when flashing using uniflash and when program using CCS this happen. can you help me to solve this problem
You can try “On connect, erase user code and unlock the device” option.
Pingback: Tinkering TI MSP430F5529 – gStore
Hello
I am doing project of msp430g2553 interface(using i2c communication) with temp 100(temperature sensor) and try to read the temperature in dispaly(16*2) but didn’t get the out put (using code composer studio) can u share me any example code for this project
Thank you sir,
Which sensor? Did you use pullup resistors for SDA-SCL pins?
Where is lcd_print.h?
All files and docs are here:
https://libstock.mikroe.com/projects/view/3233/tinkering-ti-msp430f5529
You want the truth? TI makes and sell “underpowered micros”, you know? Low everything, not only the power but also peripherals. So the price is not justified.
Otherwise, if I’ll move there, I’ll introduce them to my small hobby projects – there are still some advantages.
I may even make a visual configuration tool of my own for them…
Yeah the prices of TI products are higher than other manufacturers but I don’t think the hardware peripherals are inferior.
Not inferior but in not enough numbers compared to STM32.
True